This is geared towards the newly initiated in the world of Arduino, but I hope others will stop and take a read as well.
Here I am going to use a simple project to cover this subject. I have an Arduino Nano and 8x32 controlled by a HT1632C. This project is focused on the library used by the Arduino IDE and HT1632.
While this is a well put together library, there were a couple of items I had to check on and wanted to share some of the knowledge to help and inspire others. A note to all of the real engineers and programmers out there, I welcome constructive criticism and corrections. I want to help some other soul out there who is just dying to get a project put together but can't find quite all the information they need.
At this point I am going to make a note that the data sheet of any device / chip will provide some very useful details. I highly recommend you take a look. Even if you don't know what everything means, it is another place to start learning; but that is for another article.
What is a Library?
According to the Arduino site, the definition of a library is
"Arduino Libraries
Libraries are files written in C or C++ (.c, .cpp) which provide your sketches with extra functionality (e.g. the ability to control an LED matrix, or read an encoder, etc.)."
Within these files lies all of the information needed to grasp control of said device. Most of libraries will have a decent README file laying out how to setup the program, the device, options available, and a word or two of advice. Others might be short on the README but long on the comments in the examples. And others might leave you high and dry and your kind of left poking around to find what you can. This guide will hopefully get you started on that path if need be; or if you are just a curious mind and need to know more.
Included in an usual Arduino library are the following files:
- device_name.h - the header file. This is the file declared in the "#include" statement. And what can be done with the device.
- device_name.cpp - the source file. This is the code the C compiler works with. The .h file is not used directly, but is copied if "#included" in the .cpp file.
- keywords.txt - commands for programming and Arduino IDE coloring of class and member
- README - a little or a lot of info
- examples directory - a quick look at what it can do
Let's get wired
Now here is how the HT1632 panel is wired to the Arduino. In this case I have used a Nano, but the pins assignments are the same. Up to 4 panels are supported, but being I only have one on hand here it is.
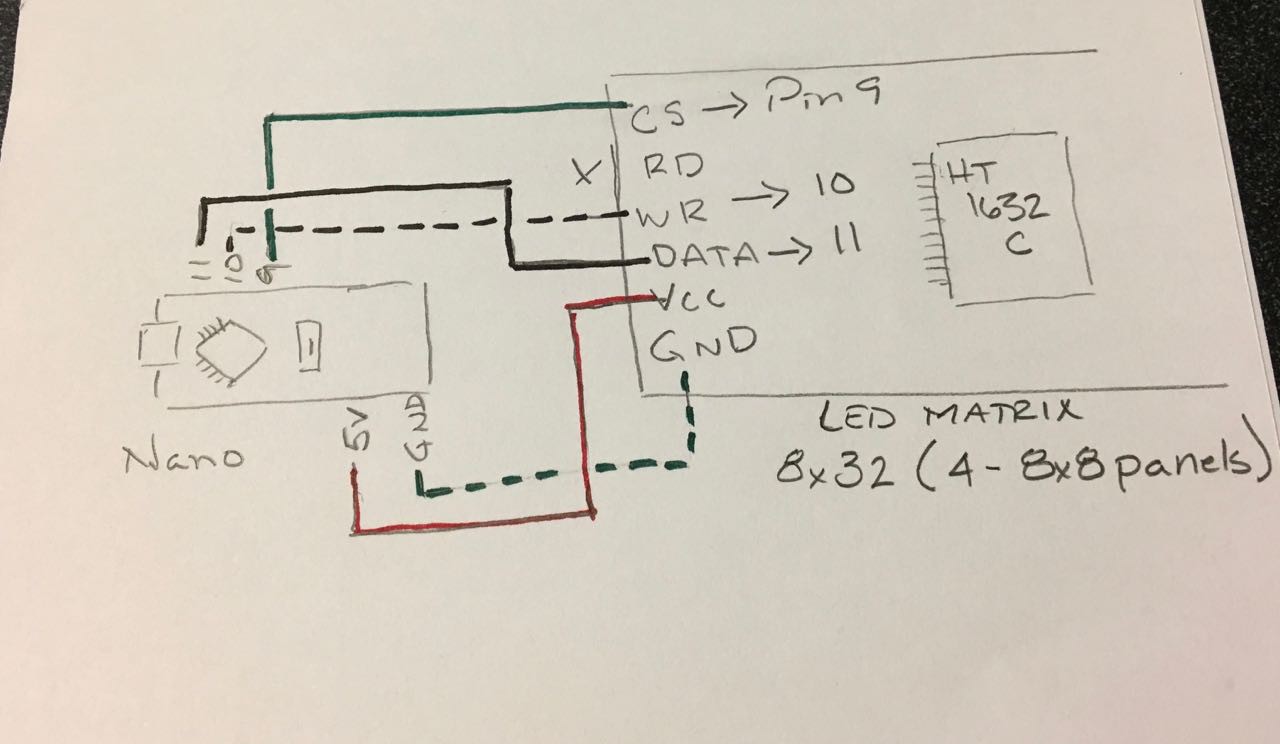
The core of the matter
Off the bat, here is the scoop. To complete this project you must have the Arduino IDE installed.
Download the library HT1632-for-Arduino. One can either download the library from GitHub through a web browser with the link above, or if you have GitHub installed on your computer that can be used as well.
Install the library in the Arduino IDE. From the menu Sketch -> Include Library -> Add .ZIP library. Once the selection window appears, navigate to where the .zip file is, select it, and click Choose.
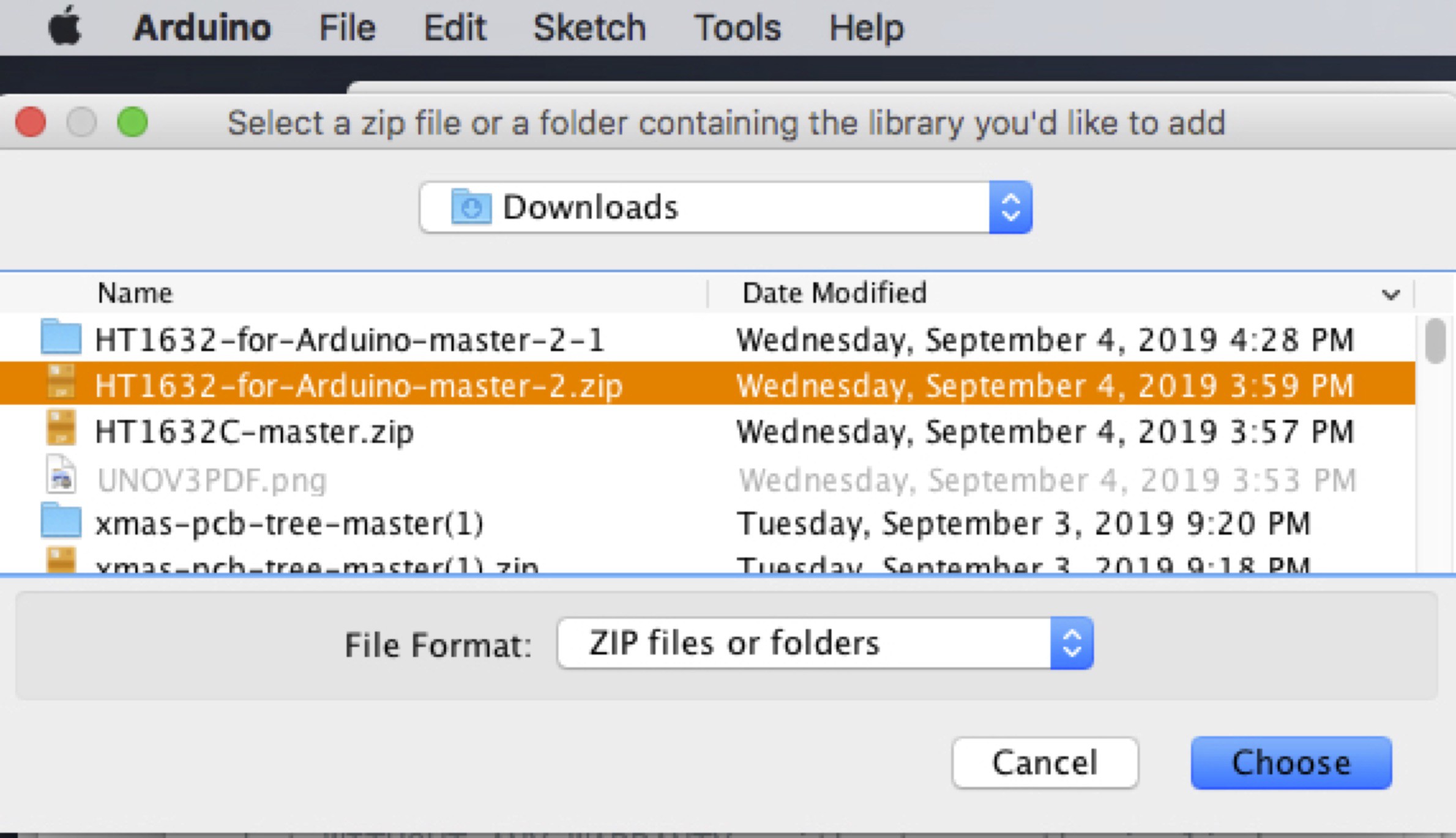
This library is a bit different than some as it must be edited to declare what type of display is being used. This edit is done in the HT1632.h file. It includes instructions on how to edit the file for your panel. Be and sure to edit the file in the library directory being used. This can be done with any text editor. I used BBEdit to make sure it is set to:
// SureElectronics 32X08 Monochrome LED Dot Matrix Unit Board
#define TYPE_3208_MONO 1
In the Arduino IDE, open the example sketch from the File menu.
File -> Examples -> HT1632-for-Arduino-master -> HT1632-Text
#include <HT1632.h>
#include <font_5x4.h>
#include <images.h>
int i = 0;
int wd;
char disp [] = "Hello, how are you?";
void setup () {
HT1632.begin(12, 10, 9);
wd = HT1632.getTextWidth(disp, FONT_5X4_END, FONT_5X4_HEIGHT);
}
void loop () {
HT1632.drawTarget(BUFFER_BOARD(1));
HT1632.clear();
HT1632.drawText(disp, OUT_SIZE - i, 2, FONT_5X4, FONT_5X4_END, FONT_5X4_HEIGHT);
HT1632.render();
i = (i+1)%(wd + OUT_SIZE);
delay(100);
}
The coloring is a little different than the Arduino IDE, but it is the same code. Let's take a look. You can use the pins that are already in the example sketch, or others as well. In my final version it uses 9, 10, 11.
Also notice that it is pretty light on comments, like a helium ballon. Personally in a situation like this I will start to comment the program myself. First with everything I know, even the simple things. If I can't figure it out I will take a peak in the .h file to see what I can glean.
And here is my first tip. If one didn't know where pins 12, 10, and 9 go, as the program doesn't really say what each pin is for. By looking in the HT1632.h file there is a function for begin and with it can see what each pin is associated with.
void begin(uint8_t pinCS1, uint8_t pinWR, uint8_t pinDATA);
(CS1, WR, DATA) pins on the panel go to pins (12, 10, 9) on the Arduino.
Here is a quick breakdown of the example:
- #include - the libraries that are required for the application.
- int and char - the variables that are going to be used.
- setup - get all the ducks in a row
- loop - let the ducks waddle and waddle and waddle until/if the loop ends
In the Arduino IDE set it up for the 328 board being used. Since this case is a Nano, select menu Tools -> Board-> *pick_your_board*. From the Tools menu setup the Port that is it connected to. It you don't see anything check if the board is connected. Do you have the USB drivers installed? Are the preferences setup to access the board files?
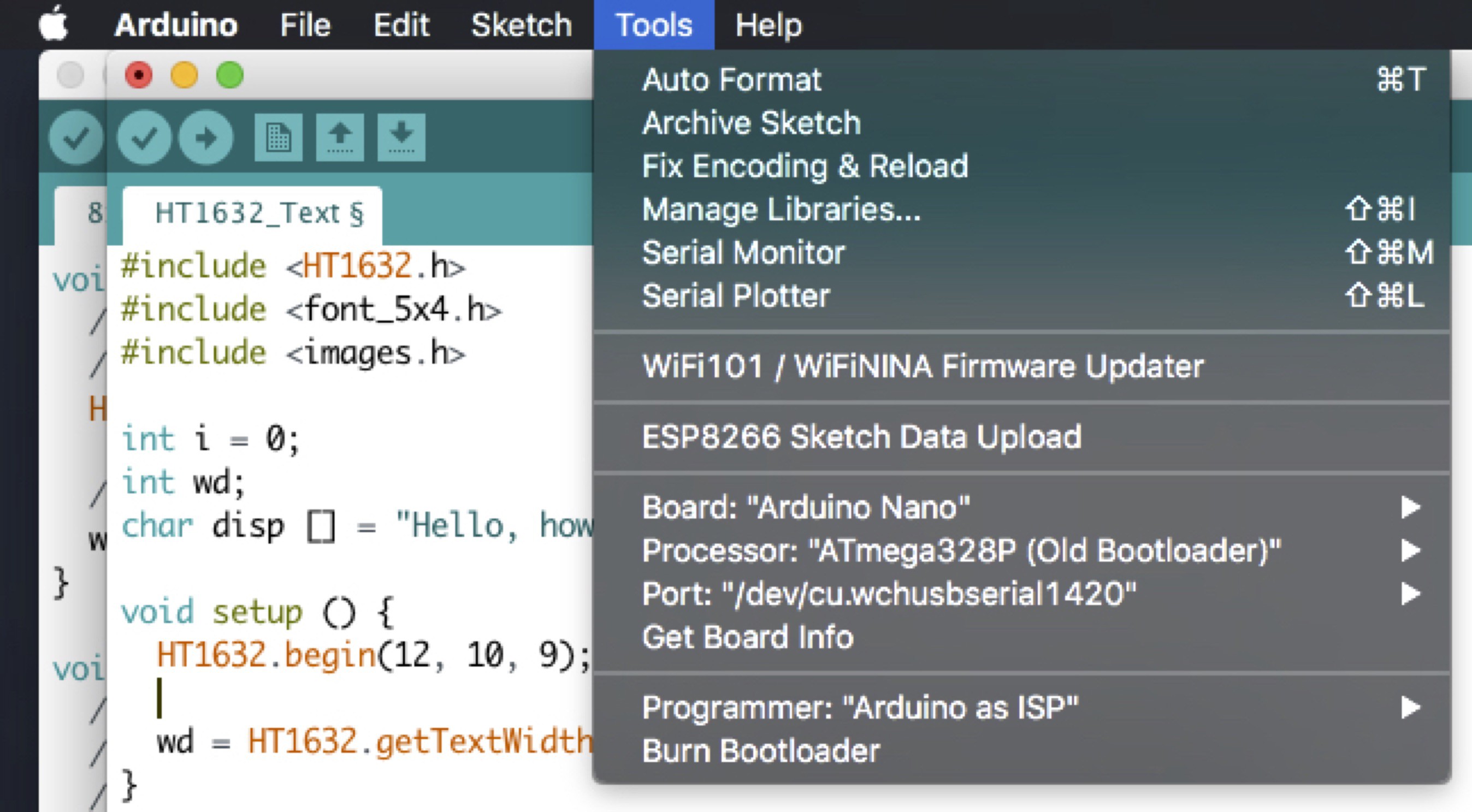
Now let's check and see if the program will compile.
From the Sketch menu select Verify/Compile.
Huh, notice an error right off the bat. There is an issue with the compiling, and probably complaining about HT1632.drawTarget(BUFFER_BOARD(1)). HT1632.drawTarget can be broken down as such:
HT1632 is the class for the device. The drawTarget is the member of the class. Inside the parens holds the value(s).
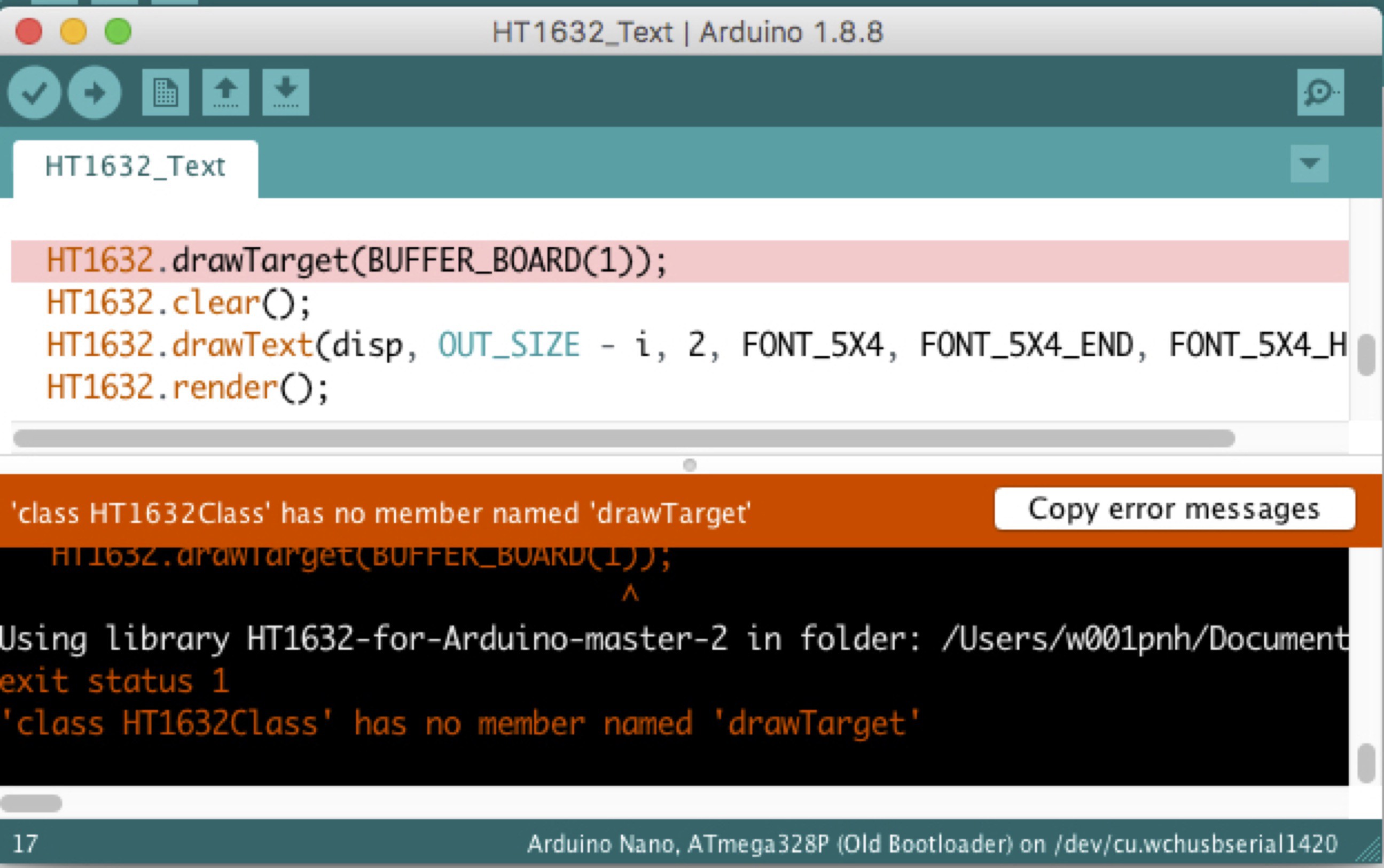
So what does one do?
The first thing to notice, and could have noticed before compiling, is that the text following HT1632. is black while the rest of them below are orange. This is the first clue as this indicates that the IDE doesn't know what member drawTarget is in conjunction with class HT1632.. The member drawTarget is from an older version of this library and has been replace.
Looking in the library directory there is a file called keywords.txt. This is extremely helpful as it tells you what commands are allowed when working with a device. It also aids the Arduino IDE color code a program. By opening up the keyword.txt file in the HT1632 library directory there is no entry for drawTarget, but there is one for renderTarget. Let's give HT1632.renderTarget(1) a try.
Shazaam! It Lives!!
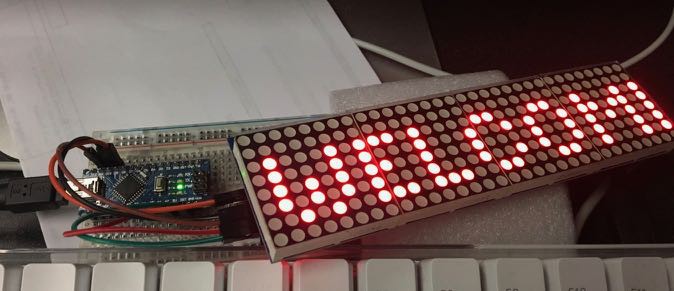
What about those variables?
In the example there are some variables that are used: FONT_5x4, FONT_5x4_END, etc. That are not declared or given a value in the start of the program, so where do they come from? This is where some curiosity comes in handy. I know that it deals with the font being used and that it is included in the beginning of the app. Going into the HT1632 library directory there is a file there called font_5x4.h. I opened it up in BBEdit to take a look at it. And in there the variables are setup for the font. The application gets the FONT_ variables from this file.
From here, take it away. Just because I can and I have the spare parts, I am pondering adding an ESP-01 and allow people to post messages to the board. I could maybe repurpose my clock and make it bigger.
As I go along I plan on coming back here to edit and update; this is version 1.0.. I have included the code I put together while playing around with the panel. It started life as the example in the library.