After clean my house, I had some pine pallets left and tried to think for recycle them.
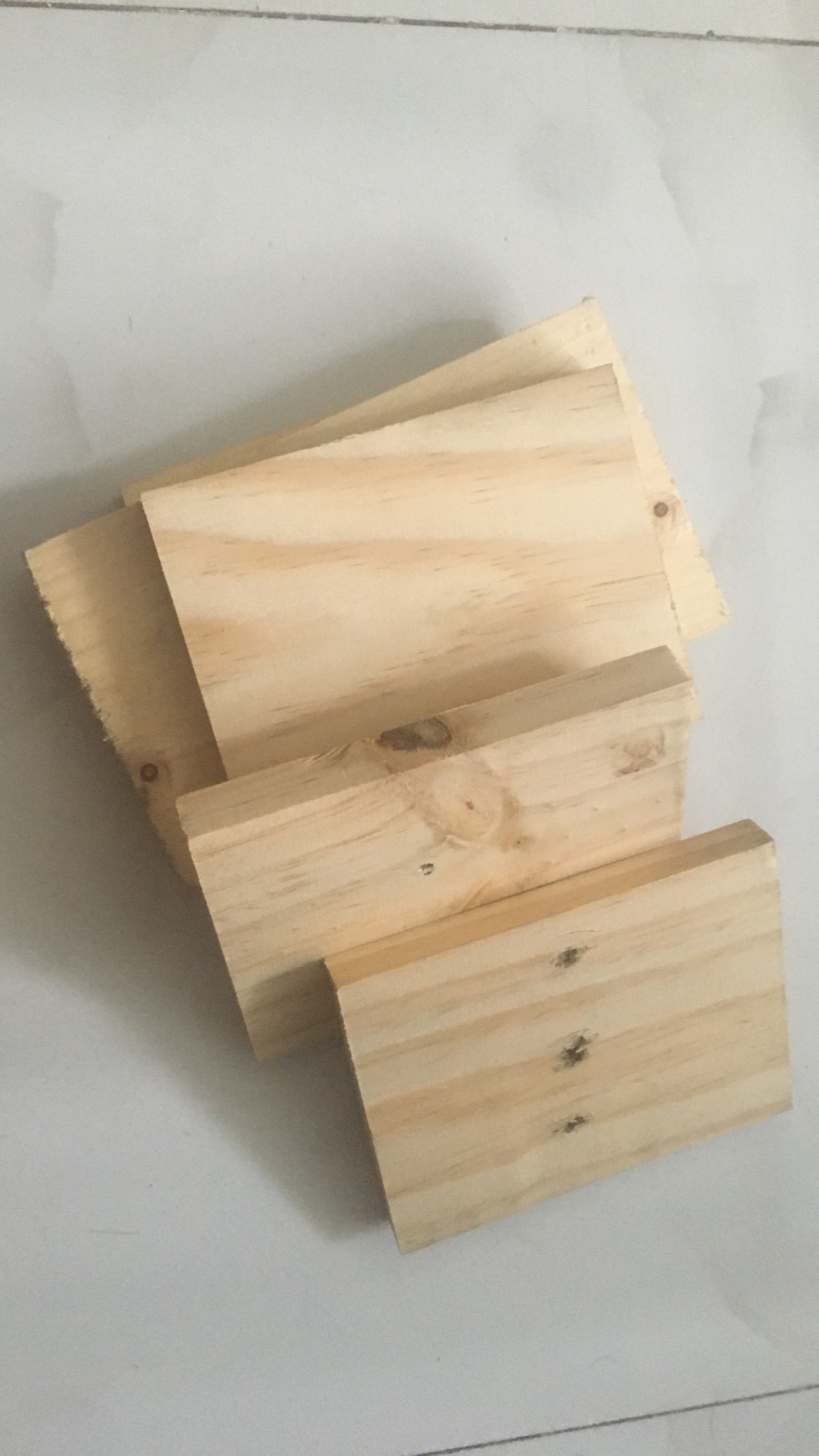
Here is a electronic diagram that I follow from: https://circuitjournal.com/50kg-load-cells-with-HX711
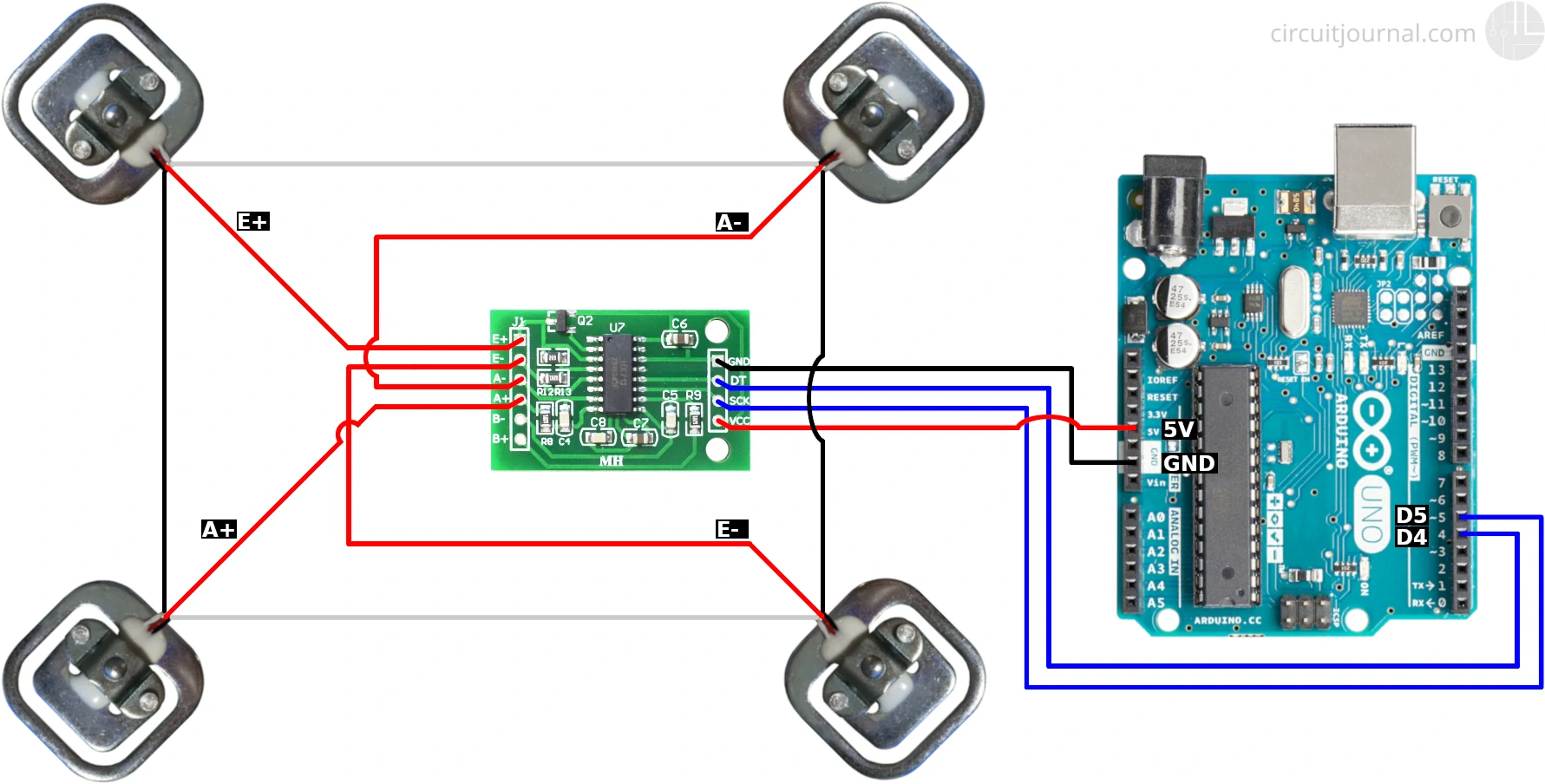
But I used Wemos D1 Mini and Oled 1306 instead Arduino Uno:
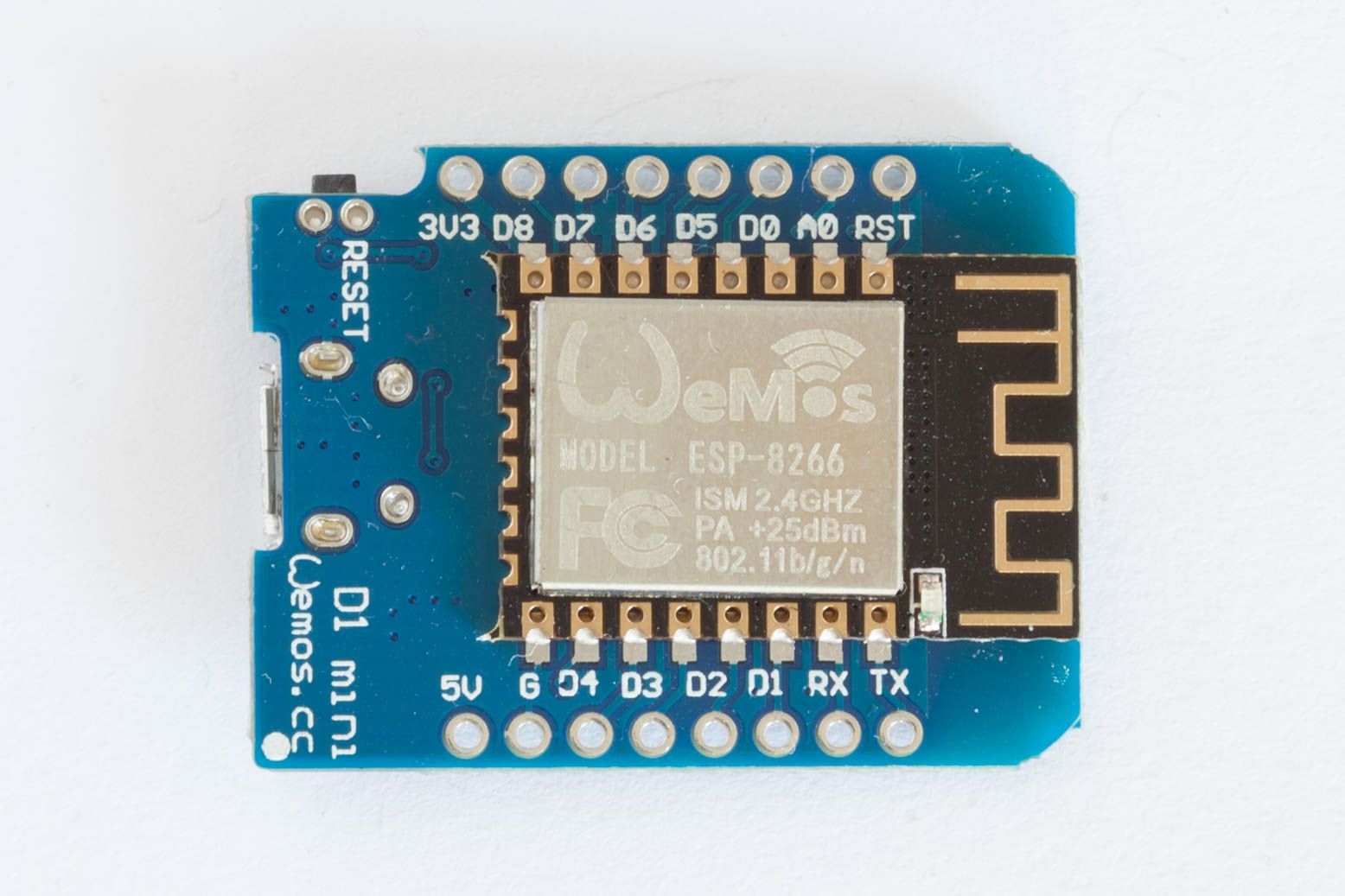
Also changed sketch for this boards. Here is wiring diagram:
OLED->WEMOS D1 MINI: VCC:3V3; GND:G; SCL:D1; SDA:D2
HX711->WEMOS D1 MINI: VCC:5V; GND:G; DT: D4; SCK:D3
Here is quick prototype:
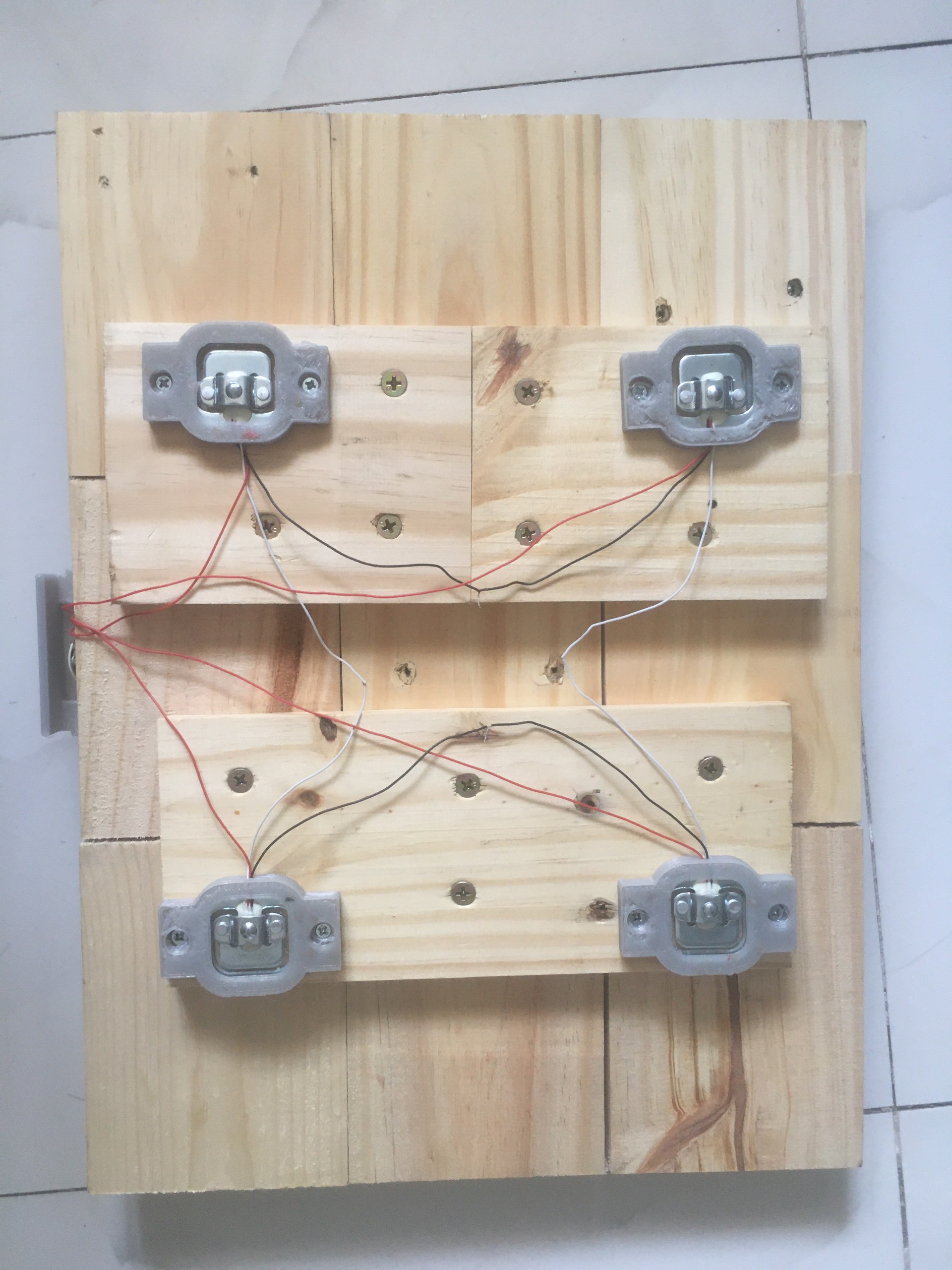
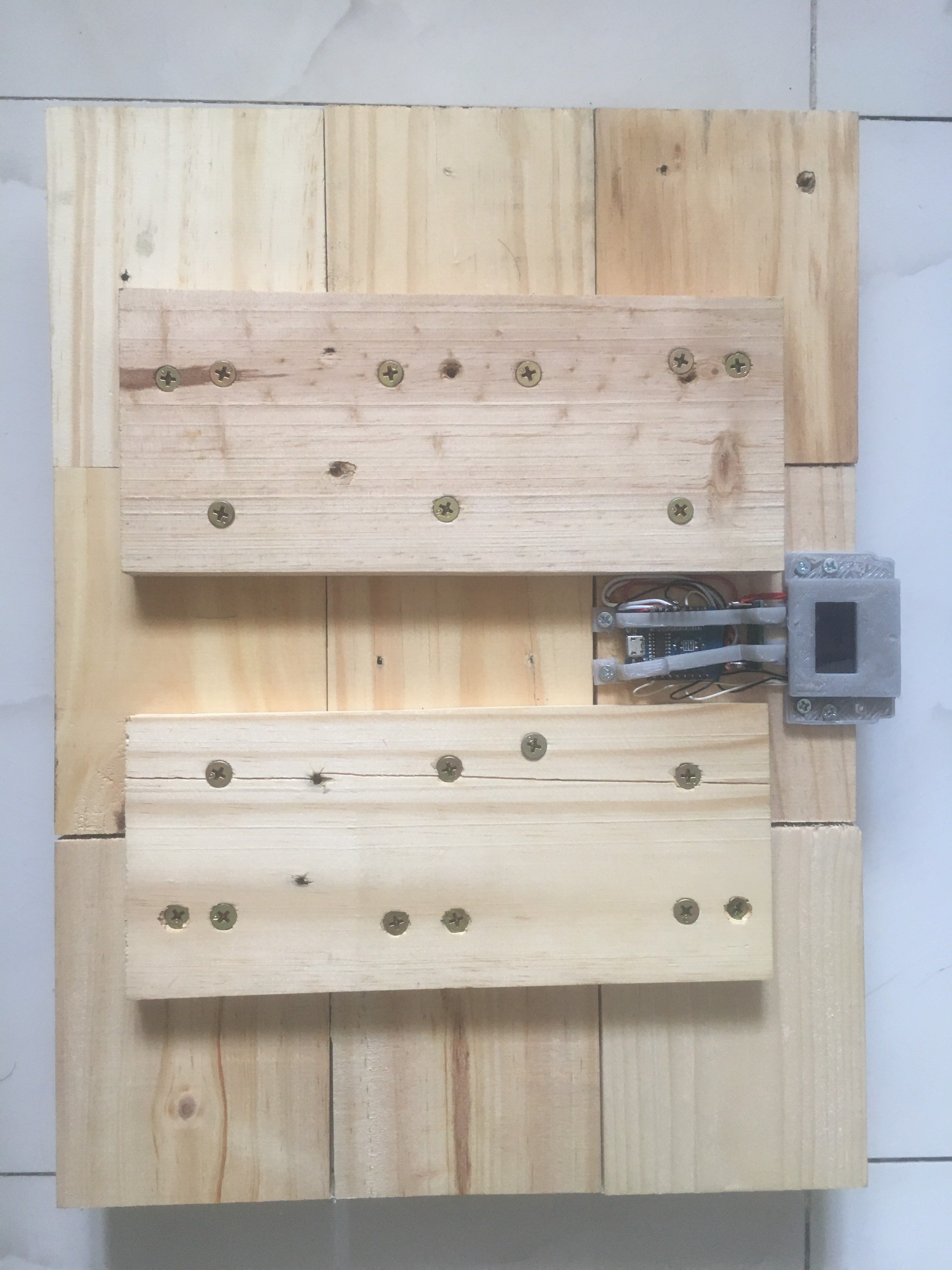
Ugly huh ?
There are some 3d printer components here:
-50kg Loadcell Bracket versionF (https://www.thingiverse.com/thing:2624188)
-Case (https://www.thingiverse.com/thing:6090225)
Before upload final sketch, check I2C adress with this code:
/*
* i2c_port_address_scanner
* Scans ports D0 to D7 on an ESP8266 and searches for I2C device. based on the original code
* available on Arduino.cc and later improved by user Krodal and Nick Gammon (www.gammon.com.au/forum/?id=10896)
* D8 throws exceptions thus it has been left out
*
*/
#include <Wire.h>
void setup() {
Serial.begin(115200);
while (!Serial); // Leonardo: wait for serial monitor
Serial.println("\n\nI2C Scanner to scan for devices on each port pair D0 to D7");
scanPorts();
}
uint8_t portArray[] = {16, 5, 4, 0, 2, 14, 12, 13};
//String portMap[] = {"D0", "D1", "D2", "D3", "D4", "D5", "D6", "D7"}; //for Wemos
String portMap[] = {"GPIO16", "GPIO5", "GPIO4", "GPIO0", "GPIO2", "GPIO14", "GPIO12", "GPIO13"};
void scanPorts() {
for (uint8_t i = 0; i < sizeof(portArray); i++) {
for (uint8_t j = 0; j < sizeof(portArray); j++) {
if (i != j){
Serial.print("Scanning (SDA : SCL) - " + portMap[i] + " : " + portMap[j] + " - ");
Wire.begin(portArray[i], portArray[j]);
check_if_exist_I2C();
}
}
}
}
void check_if_exist_I2C() {
byte error, address;
int nDevices;
nDevices = 0;
for (address = 1; address < 127; address++ ) {
// The i2c_scanner uses the return value of
// the Write.endTransmisstion to see if
// a device did acknowledge to the address.
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0){
Serial.print("I2C device found at address 0x");
if (address < 16)
Serial.print("0");
Serial.print(address, HEX);
Serial.println(" !");
nDevices++;
} else if (error == 4) {
Serial.print("Unknow error at address 0x");
if (address < 16)
Serial.print("0");
Serial.println(address, HEX);
}
} //for loop
if (nDevices == 0)
Serial.println("No I2C devices found");
else
Serial.println("**********************************\n");
//delay(1000); // wait 1 seconds for next scan, did not find it necessary
}
void loop() {
}
I modified sketch for my new wiring diagram:
/**
* Complete project details at https://RandomNerdTutorials.com/esp8266-load-cell-hx711/
*
* HX711 library for Arduino - example file
* https://github.com/bogde/HX711
*
* MIT License
* (c) 2018 Bogdan Necula
*
**/
#include <Arduino.h>
#include "HX711.h"
#include <Wire.h> // Only needed for Arduino 1.6.5 and earlier
#include "SSD1306Wire.h" // legacy: #include "SSD1306.h"
// Initialize the OLED display using Arduino Wire:
SSD1306Wire display(0x3C, SDA, SCL); // ADDRESS, SDA, SCL - SDA and SCL usually populate automatically based on your board's pins_arduino.h e.g. https://github.com/esp8266/Arduino/blob/master/variants/nodemcu/pins_arduino.h
// HX711 circuit wiring
const int LOADCELL_DOUT_PIN = 2;//D4
const int LOADCELL_SCK_PIN = 0;//D3
HX711 scale;
int reading;
int lastReading;
void setup() {
Serial.begin(115200);
// Initialising the UI will init the display too.
display.init();
display.flipScreenVertically();
display.setFont(ArialMT_Plain_10);
drawProgressBarDemo();
scale.begin(LOADCELL_DOUT_PIN, LOADCELL_SCK_PIN);
scale.set_scale(24.91974);
//scale.set_scale(-471.497); // this value is obtained by calibrating the scale with known weights; see the README for details
scale.tare(); // reset the scale to 0
}
void drawProgressBarDemo() {
for (int counter=0; counter<500; counter++)
{
int progress = (counter...
Read more »