MachiKania type P is an open platform that runs BASIC program on Raspberry Pi Pico ($4!). It is connected to 320x240 LCD and MMC/SD card, so it can read files in the card and displays the result of BASIC program execution on the LCD.
The source code and ZIP archive package are available in GitHub page ( https://github.com/machikania/phyllosoma ).
The BASIC isn't an interpreter but compiler. The BASIC system (aka KM-BASIC) compiles BASIC codes to ARM Cortex-M0 native code for the fastest execution speed.
The BASIC language is compatible to the ones of the other MachiKania computers (type M, type Z, and web), so the programs are easily transported among the types.
This photo shows an example of running a game BASIC program, SPACE ALIEN (provided as a sample in ZIP package). KM-BASIC provides the enough speed to run many games written in BASIC as it generates ARM native code.
The LCD is 320x240 resolution; 256 color photo can be displayed by BASIC code (full color photo can be also shown by a small hacking).
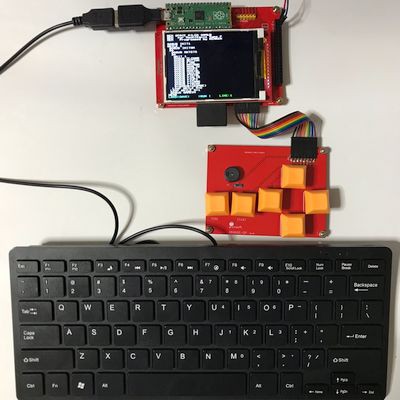
You can also connect an USB keyboard to MachiKania (photo by KenKen). The built in editor let you be able to create or edit BASIC program. Therefore, MachiKania can be also used as a stand alone PC.
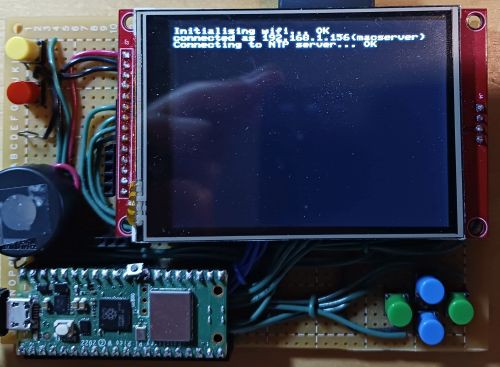
From the ver 1.3.0, MachiKania type P support the connection to internet by using Raspberry Pi Pico W. When connected to NTP server, real time clock (RTC) can be also used. Two useful classes in library make the connection to internet easy: WGET and HTTPD, used as client and server, respectively.
The syntax of BASIC language is described in help-e.txt file in documents folder of ZIP archive (repository: https://raw.githubusercontent.com/machikania/phyllosoma/main/documents/help-e.txt). The help file is translated by computer from Japanese one. Therefore, if there are somethings wrong, or confusing, please let me know.
The BASIC language supports following programing styles:
- classical line number BASIC
- structured BASIC
- object oriented BASIC (class based)
For the detail of object oriented programing, please refer class-e.txt in document folder (https://raw.githubusercontent.com/machikania/phyllosoma/main/documents/class-e.txt).
MachiKania type P can be used as not only a game console but also an I/O controller. By simple BASIC codes, one can control SPI, I2C, UART, PWM, and A/D converter.
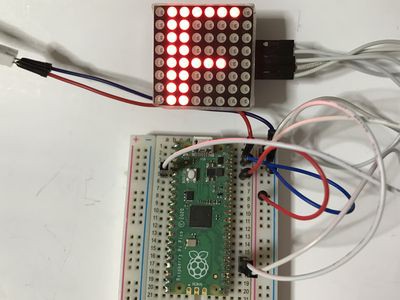
REM SPI LED Matrix x8 (MAX7219 LED Driver) SPI 10000,16,0:REM 10MHz/16bit/Mode0 REM Not Test Mode D=$0F00:SPIWRITE D,D,D,D,D,D,D,D REM Not Shutdown Mode D=$0C01:SPIWRITE D,D,D,D,D,D,D,D REM No Decode Mode D=$0900:SPIWRITE D,D,D,D,D,D,D,D REM Set Briteness D=$0A05:SPIWRITE D,D,D,D,D,D,D,D REM Scan All LEDs D=$0B07:SPIWRITE D,D,D,D,D,D,D,D DIM F(63) RESTORE FONTDT FOR I=0 TO 63 F(I)=CREAD() NEXT FOR Y=0 TO 7 D=(Y+1)<<8 SPIWRITE D+F(Y),D+F(8+Y),D+F(16+Y),D+F(24+Y),D+F(32+Y),D+F(40+Y),D+F(48+Y),D+F(56+Y) NEXT END LABEL FONTDT CDATA $38,$6C,$C6,$FE,$C6,$C6,$C6,$00:REM A CDATA $FC,$66,$66,$7C,$66,$66,$FC,$00:REM B CDATA $3C,$66,$C0,$C0,$C0,$66,$3C,$00:REM C CDATA $F8,$6C,$66,$66,$66,$6C,$F8,$00:REM D CDATA $FE,$C0,$C0,$F8,$C0,$C0,$FE,$00:REM E CDATA $FE,$C0,$C0,$F8,$C0,$C0,$C0,$00:REM F CDATA $3C,$66,$C0,$CE,$C6,$66,$3C,$00:REM G CDATA $C6,$C6,$C6,$FE,$C6,$C6,$C6,$00:REM H
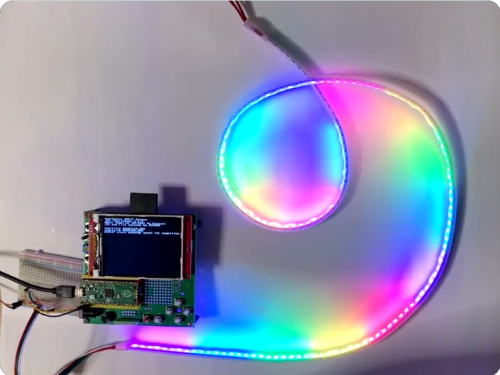
Photo is an example of controling LED-tape (containing 144 LEDs) by MachiKania type P (programmed by KenKen). For a movie, please refer this page.
Following is an example to connect to internet. Here, the BASIC program uses WGET and JSON classes. Raspberry Pi Pico W is used.
useclass JSON,WGET
usevar Min0,Max0,Fstr0
usevar Min1,Max1,Fstr1
if val(strftime$("%Y"))<2023 then ntp
j$=WGET::FORSTRING$("https://www.recfor.net/projects/weather/tokyo.php")
REM j$=WGET::FORSTRING$("https://www.recfor.net/projects/weather/osaka.php")
REM Forecast of any city can be obtained by AccuWeather service.
o=new(JSON,j$)
Min0#=o.FQUERY#(".DailyForecasts[0].Temperature.Minimum.Value")
Max0#=o.FQUERY#(".DailyForecasts[0].Temperature.Maximum.Value")
Fstr0$=o.SQUERY$(".DailyForecasts[0].Day.IconPhrase")
Min1#=o.FQUERY#(".DailyForecasts[1].Temperature.Minimum.Value")
Max1#=o.FQUERY#(".DailyForecasts[1].Temperature.Maximum.Value")
Fstr1$=o.SQUERY$(".DailyForecasts[1].Day.IconPhrase")
print "Today is ";strftime$("%m/%d (%a)")
print "Today's forecast is ";Fstr0$
print "Minimum temperature is ";Min0#;" degree-C"
print "Maximum temperature is ";Max0#;" degree-C"
print "Tomorrow's forecast is ";Fstr1$
print "Minimum temperature is ";Min1#;" degree-C"
print "Maximum temperature is ";Max1#;" degree-C"
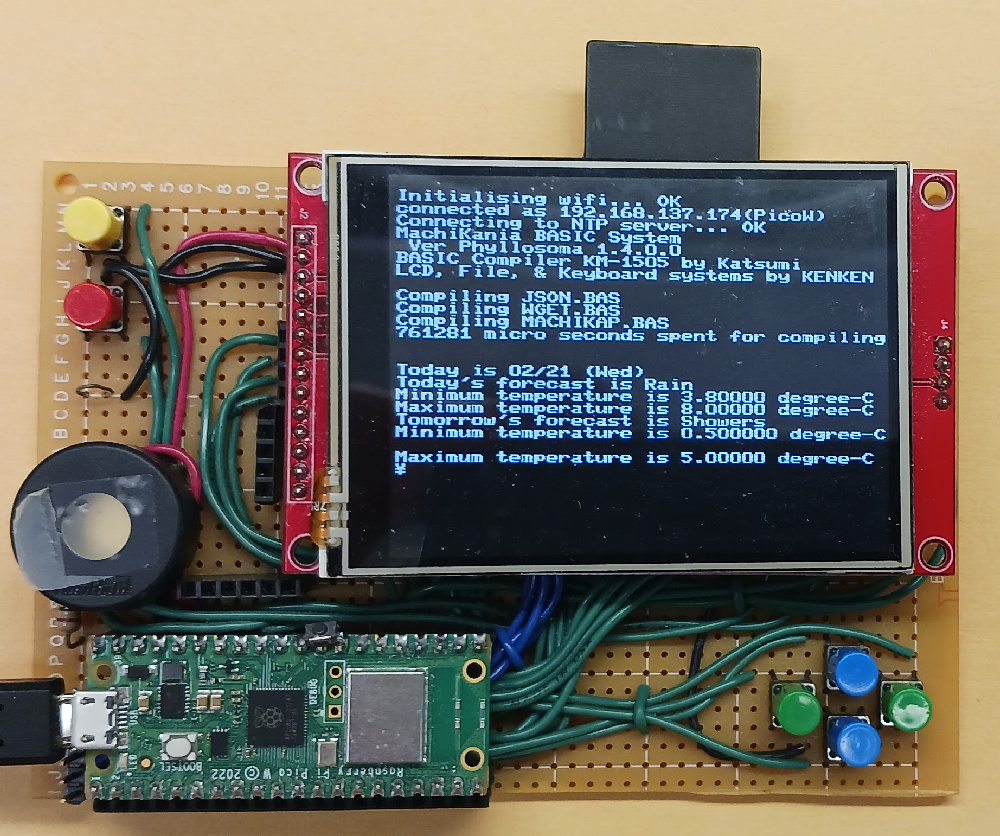
Following is an example to construct an http server by MachiKania:
useclass HTTPD
h=new(HTTPD,80,"/httproot")
print "server started as http://";ifconfig$(0);"/"
do
h.START()
system 201,val(h.GETPARAM$("led"))
print h.LASTURI$()
loop
Put a text file as /httproot/index.htm:
<html><head><title>Test</title>
<meta name="viewport" content="width=1, initial-scale=5"></head>
<body>
<h5 style="text-align:center"><a href="?led=1">ON</a></h5>
<h5 style="text-align:center"><a href="?led=0">OFF</a></h5>
</body></html>
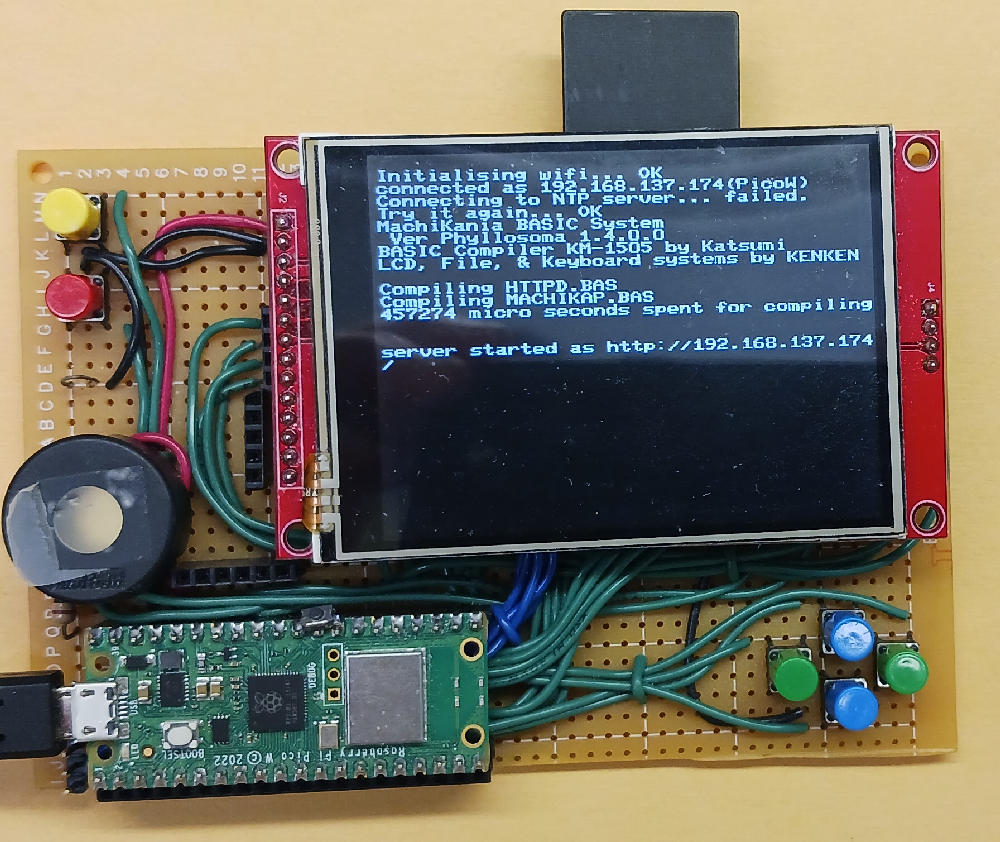
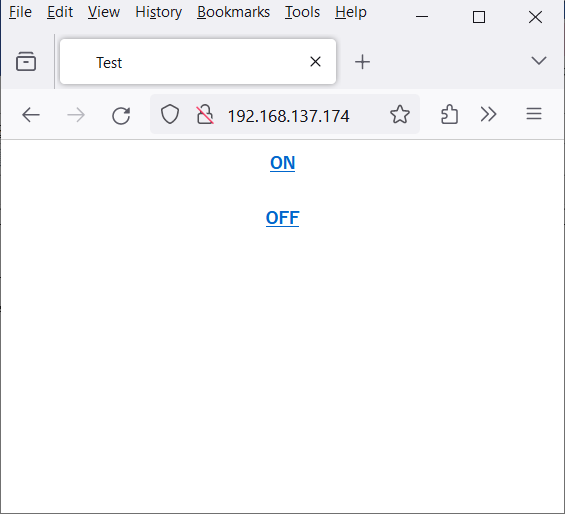
You can contol the LED on Raspberry Pi Pico W by clicking "ON" and "OFF" link on browser.
To control I/O, the LCD and MMC/SD card aren't necessary. One can embed BASIC program to MachiKania type P and install to naked Raspberry Pi Pico (photo by KenKen), For the detail, please refer embed-e.txt in documents folder (https://raw.githubusercontent.com/machikania/phyllosoma/main/documents/embed-e.txt) and my blog page (google translated).