As mentioned before in my last project log, I used the long silence to dive deeper in the electronics of the Polo 6R.
In this project log I want to share some details regarding the Steering Column Switch (or in German “Lenkstockschalter”).
Its Task is to measure the rotation position of the steering wheel and it provides the switches for wipers, turning signal and light options.
A while ago I bought an old steering column switch from eBay as well as the case for it. I also bought a key switch for usage as a ignition lock.
Here’re some photos:
What I wanted to build was an USB interface which connects to the PC running the simulation game. That way you could control the corresponding functions with the simulation game.
I used an Arduino micro which can use USB HID profiles, so that it will be recognized as a keyboard by my PC.
Now my task was to find out the pin assignments for the column switch, which you can find below.
If you want to build this part of the project as well, here’s the Arduino sketch and the wiring diagram for it.
/*
VW Polo 6R - Lenkstockschalter - Controller Software
by Leon Bataille
Version 1.0
Last modified: 04.07.2017
Pin assignments:
A0: HW (FSW Wischwasser)
A1: 53c (HSW)
A2: (R) (MFA OK/Reset)
A3: I (SW Intervallschaltung)
A4: 1 (SW Wischer-Stufe 1)
A5: 2 (SW Wischer-Stufe 2)
D2: SDA - I2C Arduino Communication
D3: SCL - I2C Arduino Communication
D4: L (Blinker links <-)
D5: R (Blinker rechts ->)
D6: 30 (Klemme 30: Lichthupe)
D7: 56 (Klemme 56: Fernlicht)
D8: 7 (GRA Reset/+)
D9: 8 (GRA Set/-)
D10: 3 (GRA an)
D12: auf (Trip/MFA+ auf)
D13: ab (Trip/MFA+ ab)
More information on my hackaday project site:
https://hackaday.io/project/6288
*/
#include <Wire.h>
#include <Keyboard.h>
//Pin Definitions
//-Inputs
int SW_WW = A0;
int HSW = A1;
int MFA_OK = A2;
int SW_INT = A3;
int SW_lv1 = A4;
int SW_lv2 = A5;
int tisch_hoch = 0;
int tisch_runter = 1;
int blnk_l = 4;
int blnk_r = 5;
int licht_hupe = 6;
int licht_fern = 7;
int GRA_plus = 8;
int GRA_minus = 9;
int GRA_an = 10;
int MFA_auf = 11;
int MFA_ab = 12;
bool state_GRA = 0;
bool lastState_SW_WW = 0;
bool lastState_HSW = 0;
bool lastState_MFA_OK = 0;
bool lastState_SW_INT = 0;
bool lastState_SW_lv1 = 0;
bool lastState_SW_lv2 = 0;
bool lastState_blnk_l = 0;
bool lastState_blnk_r = 0;
bool lastState_licht_hupe = 0;
bool lastState_licht_fern = 0;
bool lastState_GRA_plus = 0;
bool lastState_GRA_minus = 0;
bool lastState_GRA_an = 0;
bool lastState_MFA_auf = 0;
bool lastState_MFA_ab = 0;
void setup() {
// put your setup code here, to run once:
//PinModes
//-> Alle Eingänge sind mit Pull-Up-Widerständen verbunden
//Siehe: https://electrosome.com/switch-arduino-uno/
pinMode(SW_WW, INPUT);
pinMode(HSW, INPUT);
pinMode(MFA_OK, INPUT);
pinMode(SW_INT, INPUT);
pinMode(SW_lv1, INPUT);
pinMode(SW_lv2, INPUT);
pinMode(blnk_l, INPUT);
pinMode(blnk_r, INPUT);
pinMode(licht_hupe, INPUT);
pinMode(licht_fern, INPUT);
pinMode(GRA_plus, INPUT);
pinMode(GRA_minus, INPUT);
pinMode(GRA_an, INPUT);
pinMode(MFA_auf, INPUT);
pinMode(MFA_ab, INPUT);
pinMode(tisch_hoch, OUTPUT);
pinMode(tisch_runter, OUTPUT);
Keyboard.begin();
Wire.begin();
}
void loop() {
// put your main code here, to run repeatedly:
//MFA_OK
if (digitalRead(MFA_OK) == LOW && lastState_MFA_OK == 0) //If the switch is pressed
{
lastState_MFA_OK = 1;
Keyboard.print("i");
}
else if (digitalRead(MFA_OK) == HIGH && lastState_MFA_OK == 1) //If the switch is released
{
lastState_MFA_OK = 0;
}
//SW_lv1
if (digitalRead(SW_lv1) == LOW && lastState_SW_lv1 == 0) //If the switch is pressed
{
lastState_SW_lv1 = 1;
Keyboard.print("w");
}
else if (digitalRead(SW_lv1) == HIGH && lastState_SW_lv1 == 1) //If the switch is released
{
lastState_SW_lv1 = 0;
}
//blnk_l
if (digitalRead(blnk_l) == LOW && lastState_blnk_l == 0) //If the switch is pressed
{
lastState_blnk_l = 1;
Keyboard.print("y");
}
else if (digitalRead(blnk_l) == HIGH && lastState_blnk_l == 1) //If the switch is released
{
lastState_blnk_l = 0;
Keyboard.print("y");
}
//blnk_r
if (digitalRead(blnk_r) == LOW && lastState_blnk_r == 0) //If the switch is pressed
{
lastState_blnk_r = 1;
Keyboard.print("x");
}
else if (digitalRead(blnk_r) == HIGH && lastState_blnk_r == 1) //If the switch is released
{
lastState_blnk_r = 0;
Keyboard.print("x");
}
//licht_hupe
if (digitalRead(blnk_r) == LOW) //If the switch is pressed
{
Keyboard.print("j");
}
//licht_fern
if (digitalRead(licht_fern) == LOW && lastState_licht_fern == 0) //If the switch is pressed
{
lastState_licht_fern = 1;
Keyboard.print("k");
}
else if (digitalRead(licht_fern) == HIGH && lastState_licht_fern == 1) //If the switch is released
{
lastState_licht_fern = 0;
Keyboard.print("k");
}
//GRA_an
if (digitalRead(GRA_an) == LOW && lastState_GRA_an == 0) //If the switch is on
{
lastState_GRA_an = 1;
state_GRA = 1;
Wire.beginTransmission(8);
Wire.write("CCt_1");
Wire.endTransmission();
//GRA_plus
if (digitalRead(GRA_plus) == LOW && lastState_GRA_plus == 0) //If the switch is pressed
{
lastState_GRA_plus = 1;
Keyboard.print("+");
}
else if (digitalRead(GRA_plus) == HIGH && lastState_GRA_plus == 1) //If the switch is released
{
lastState_GRA_plus = 0;
}
//GRA_minus
if (digitalRead(GRA_minus) == LOW && lastState_GRA_minus == 0) //If the switch is pressed
{
lastState_GRA_minus = 1;
Keyboard.print("#");
}
else if (digitalRead(GRA_minus) == HIGH && lastState_GRA_minus == 1) //If the switch is released
{
lastState_GRA_minus = 0;
}
}//Ende GRA_an
else if (digitalRead(GRA_an) == HIGH && lastState_GRA_an == 1) //If the switch is off
{
state_GRA = 0;
Wire.beginTransmission(8);
Wire.write("CCt_0");
Wire.endTransmission();
lastState_GRA_an = 0;
}
else //GRA_aus
{
//GRA_plus
if (digitalRead(GRA_plus) == LOW && lastState_GRA_plus == 0) //If the switch is pressed
{
lastState_GRA_plus = 1;
Keyboard.print("c");
//Transmit CC Status
if (state_GRA == 0)
{
state_GRA = 1;
Wire.beginTransmission(8);
Wire.write("CCt_1");
Wire.endTransmission();
}
else
{
state_GRA = 0;
Wire.beginTransmission(8);
Wire.write("CCt_0");
Wire.endTransmission();
}
}
else if (digitalRead(GRA_plus) == HIGH && lastState_GRA_plus == 1) //If the switch is released
{
lastState_GRA_plus = 0;
}
}
//MFA_auf
if (digitalRead(MFA_auf) == LOW && lastState_MFA_auf == 0) //If the switch is pressed
{
lastState_MFA_auf = 1;
Keyboard.print("ü");
}
else if (digitalRead(MFA_auf) == HIGH && lastState_MFA_auf == 1) //If the switch is released
{
lastState_MFA_auf = 0;
}
//MFA_ab
if (digitalRead(MFA_ab) == LOW && lastState_MFA_ab == 0) //If the switch is pressed
{
lastState_MFA_ab = 1;
Keyboard.print("ä");
}
else if (digitalRead(MFA_ab) == HIGH && lastState_MFA_ab == 1) //If the switch is released
{
lastState_MFA_ab = 0;
}
}
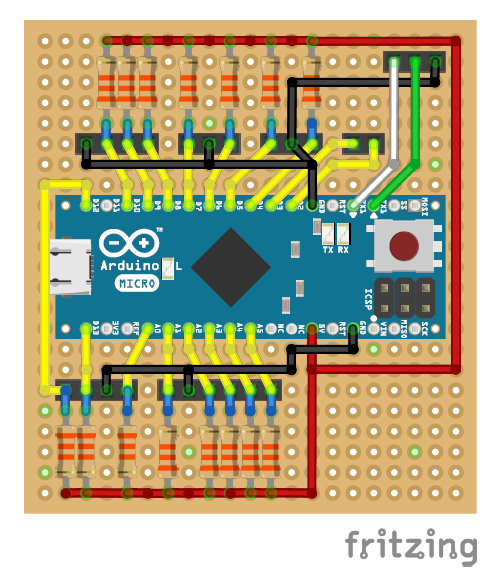
That’s it for today. The next project log will be about the new instrument cluster setup.
Thanks for your support!
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.
Wow amazing work you did here, thank you. It will help me a lot for my school project.
I sent you a private message about another thing if you can check it :)
Are you sure? yes | no