Use this code to connect a Toggle Flip Switch to an Arduin Pro Micro or Leonardo.
The pin to connect is Digital PIN 8 and GND
ON will output "a"
OFF will output "b"
Change if you like!
#include <Keyboard.h>
const byte switchPin = 8;
byte oldSwitchState = HIGH; // assume switch open because of pull-up resistor
const unsigned long debounceTime = 10; // milliseconds
void setup ()
{
Serial.begin (9600);
pinMode (8, INPUT_PULLUP);
} // end of setup
void loop ()
{
Keyboard.begin();
// see if switch is open or closed
byte switchState = digitalRead (switchPin);
// has it changed since last time?
if (switchState != oldSwitchState)
{
oldSwitchState = switchState; // remember for next time
delay (debounceTime); // debounce
if (switchState == LOW)
{
Serial.println ("Switch closed.");
Keyboard.press('a');
delay(100);
Keyboard.releaseAll();
} // end if switchState is LOW
else
{
Serial.println ("Switch opened.");
Keyboard.press('b');
delay(100);
Keyboard.releaseAll();
} // end if switchState is HIGH
} // end of state change
// other code here ...
} // end of loop
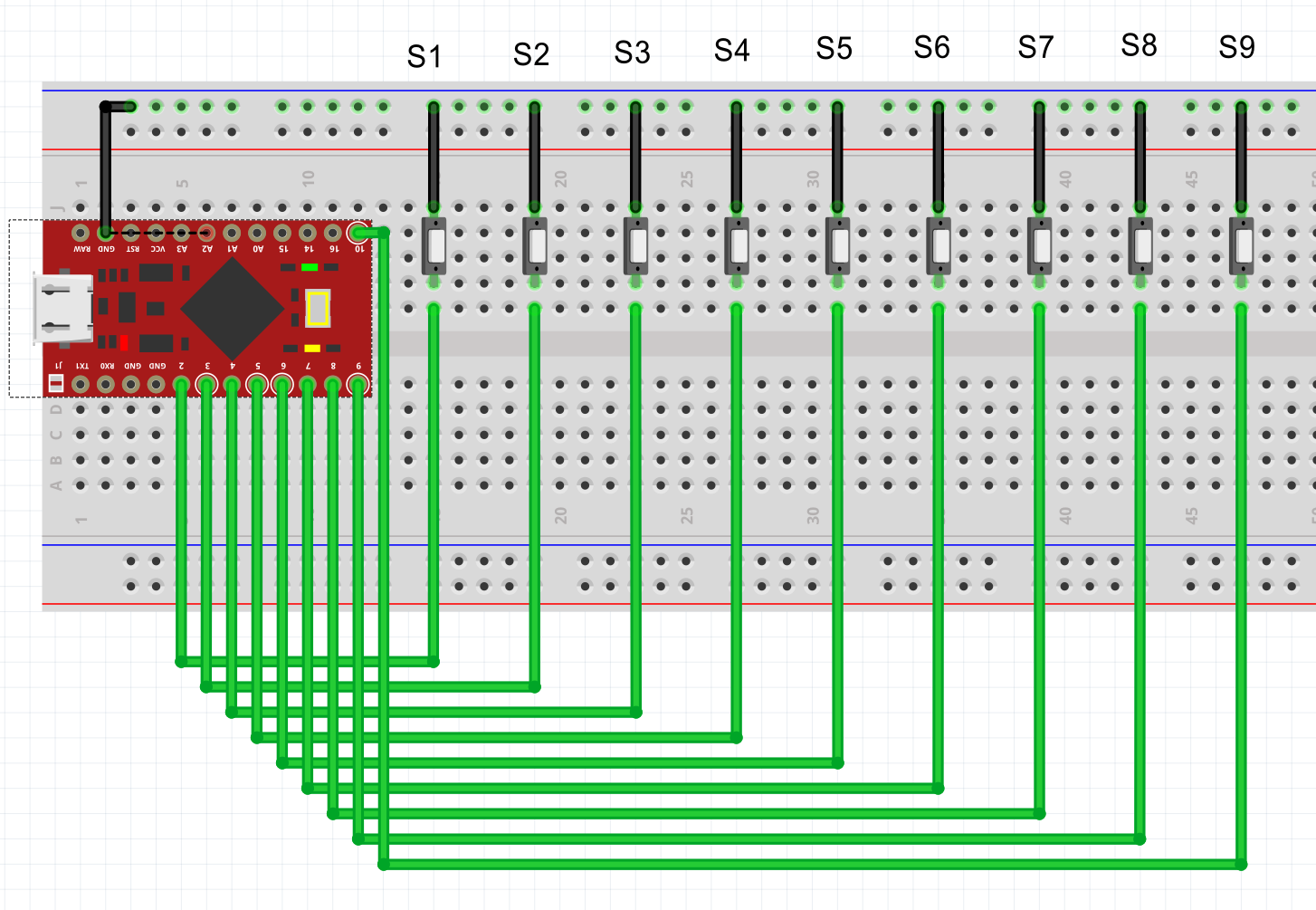
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.