Building an Interpreter
Writing micro-code for an interpreter needs to done in incremental steps to avoid possible insanity by the author (some say it is too late)! So here are the stages that I used:
- Micro-code address (addr) counter
- Basic interpreter with inline (micro-code) op-code
- Interpreter with inline (micro-code) counter
- Interpreter with inline (micro-code) counter and jmp_adr op-code.
- Interpreter with op-code inc_addr.
- Interpreter with op-code call and rtn (return) op-codes.
The micro-code address (addr) counter
Here is the micro-code:
LABEL ADR ASM COMMENT ADDR CODE COUNT 128 _0 Initalise 0X80 0X96 129 REGD 0X81 0XFB 130 _0 0X82 0X96 131 ADDR 0X83 0XF2 SHOW 132 ADDR Show Address 0X84 0XF2 133 REGA 0X85 0XFA INCREMENT 134 _1 Increment Address 0X86 0X95 135 REGP 0X87 0XF8 136 ADDR 0X88 0XF2 137 REGQ 0X89 0XF9 138 ADD 0X8A 0XF8 139 ADDR 0X8B 0XF2 DELAY 140 _255 255 MS DELAY 0X8C 0X94 141 TEMP 0X8D 0XF0 142 SHOW RETURN ADDRESS 0X8E 0X92 143 RETURN 0X8F 0XF1 144 _96 DELAY SUBROUTINE 0X90 0X93 145 JMP 0X91 0XFF 146 Const _132 0X92 0X84 147 Const _96 0X93 0X60 148 Const _255 0X94 0XFF 149 Const _1 0X95 0X01 150 Const _0 0X96 0X00
I suspect that now you understand why micro-code is so unreadable. Let us look at lines 128 and 129:
- Fetch constant "0" (located at address 150)
- Deposit in register "D" or the data register (LEDs) on the front panel.
This is how the coded needs to be analysed.
Basic interpreter with inline (micro-code) op-code
Here is the micro-code:
Label Adr Code Comment Addr Code RESET 128 _255 SET JUMP TO JMP 0X80 0XDF 129 JUMP 0X81 0XF7 130 _232 SET SP 0X82 0XDE 131 SP 0X83 0XEE 132 CODE SET PC TO START OF CODE 0X84 0XDB 133 IP 0X85 0XEF INTERP 134 IP SAVE IP 0X86 0XEF 135 POINTER 0X87 0XF6 136 IP INC IP 0X88 0XEF 137 REGP 0X89 0XF8 138 _1 0X8A 0XDD 139 REGQ 0X8B 0XF9 140 ADD 0X8C 0XF8 141 IP 0X8D 0XEF 142 _246 ^POINTER 0X8E 0XDC 143 JMP 0X8F 0XFF INLINE 144 IP 0X90 0XEF 145 JMP 0X91 0XFF CODE 146 144 &INLINE 0X92 0X90 147 NOP 0X93 0X00 ... ... ... ... 218 NOP 0XDA 0X00 219 Const _146 0XDB 0X92 220 Const _246 0XDC 0XF6 221 Const _1 0XDD 0X01 222 Const _232 0XDE 0XE8 223 Const _255 0XDF 0XFF
The interpreter is remarkably simple:
- Set the jump to the address of the value pointed to by IP
- Increment IP to the next instruction
- Make the jump
The instruction just needs to return by jumping to the interpreter.
For the inline instruction, the instruction has to recalculate the address of the next instruction and set the IP.
Interpreter with inline (micro-code) counter
Here is the micro-code:
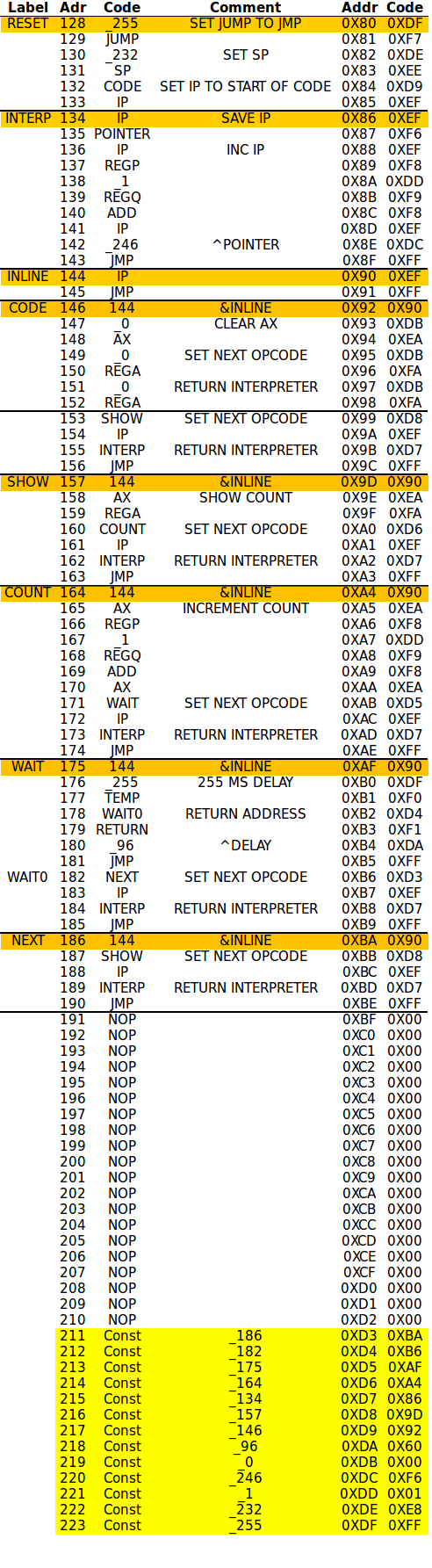
Now it is staring to look interesting, it works but it does not appear to be very space efficient.
Interpreter with inline (micro-code) counter and jmp_adr op-code
Here is the micro-code:
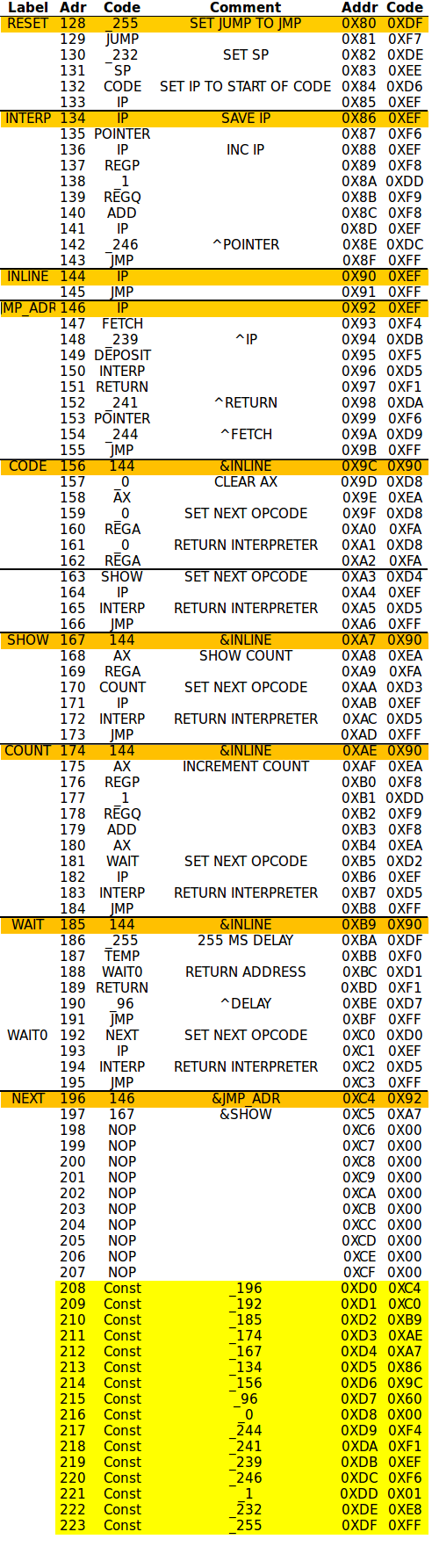
Interpreter with op-codes call/rtn/jmp/Include
Here is the micro-code:
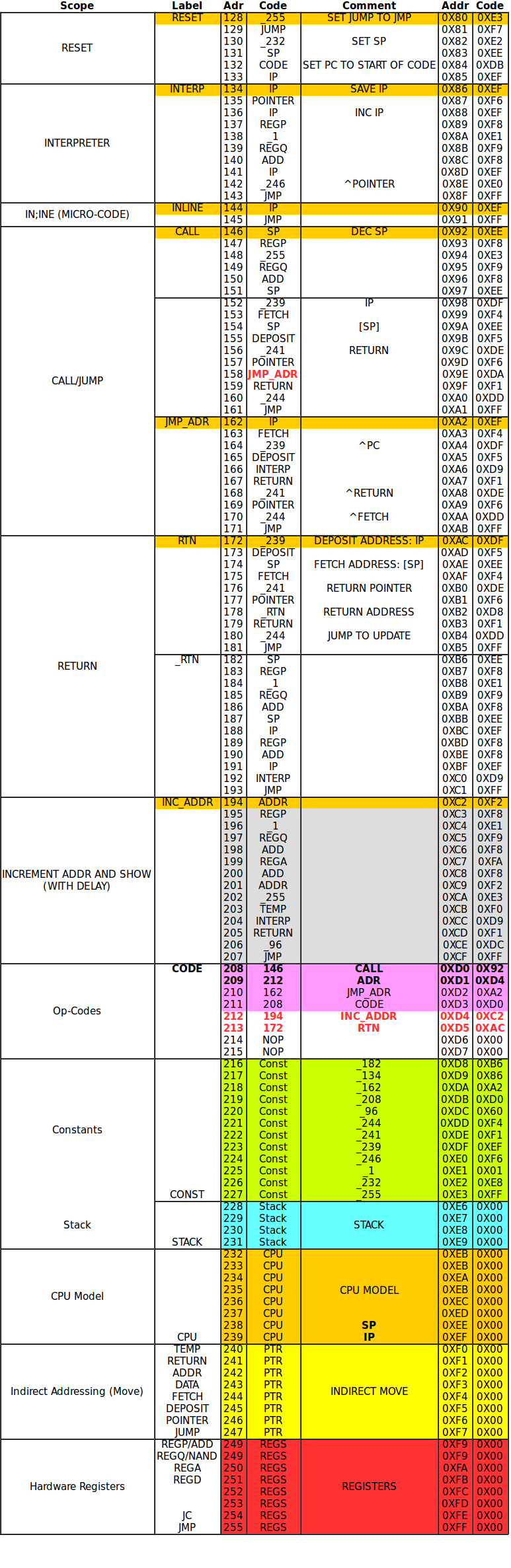
Okay, while the above micro-code just fits into the available memory, the actual op-codes are only 5 bytes:
- Call
- Addr (4)
- Jmp_Adr (1)
- Inc_Addr (front panel)
- Rtn
The executed bytes (exclude the delay loop) is 136 while the Inc_Addr only is 14 bytes. So the interpreter is about 10x slower than native TTA micro-code.
Possible Interpreter Monitor
If I improve the hardware for I/O (serial perhaps?), I may be able to fit the above interpreter into ROM on the TTA8. Failing that it is time to design the TTA16.
AlanX
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.