”MEMIDION" is MIDI instruments that you can play with DAW software or MIDI controllers.
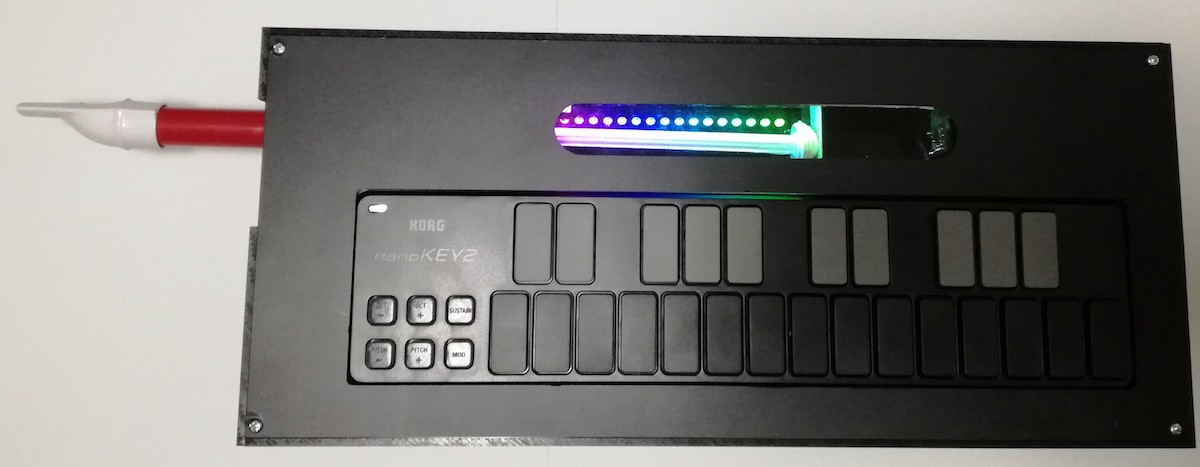
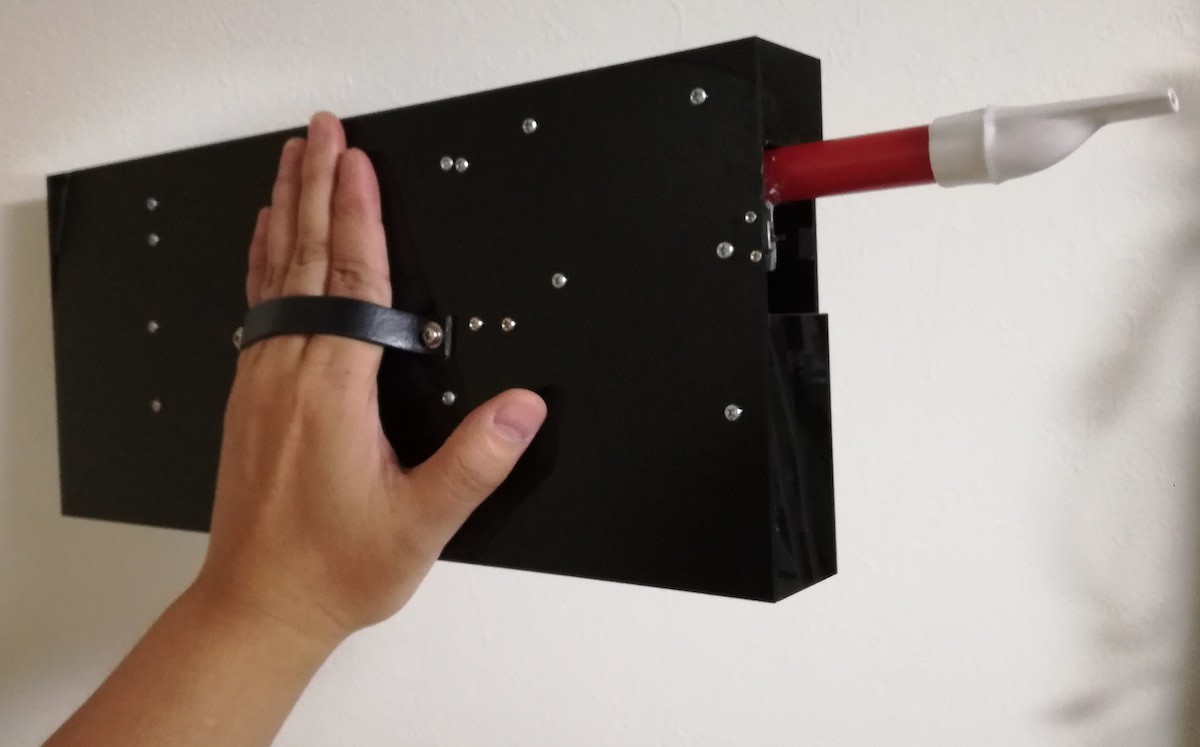
Structure
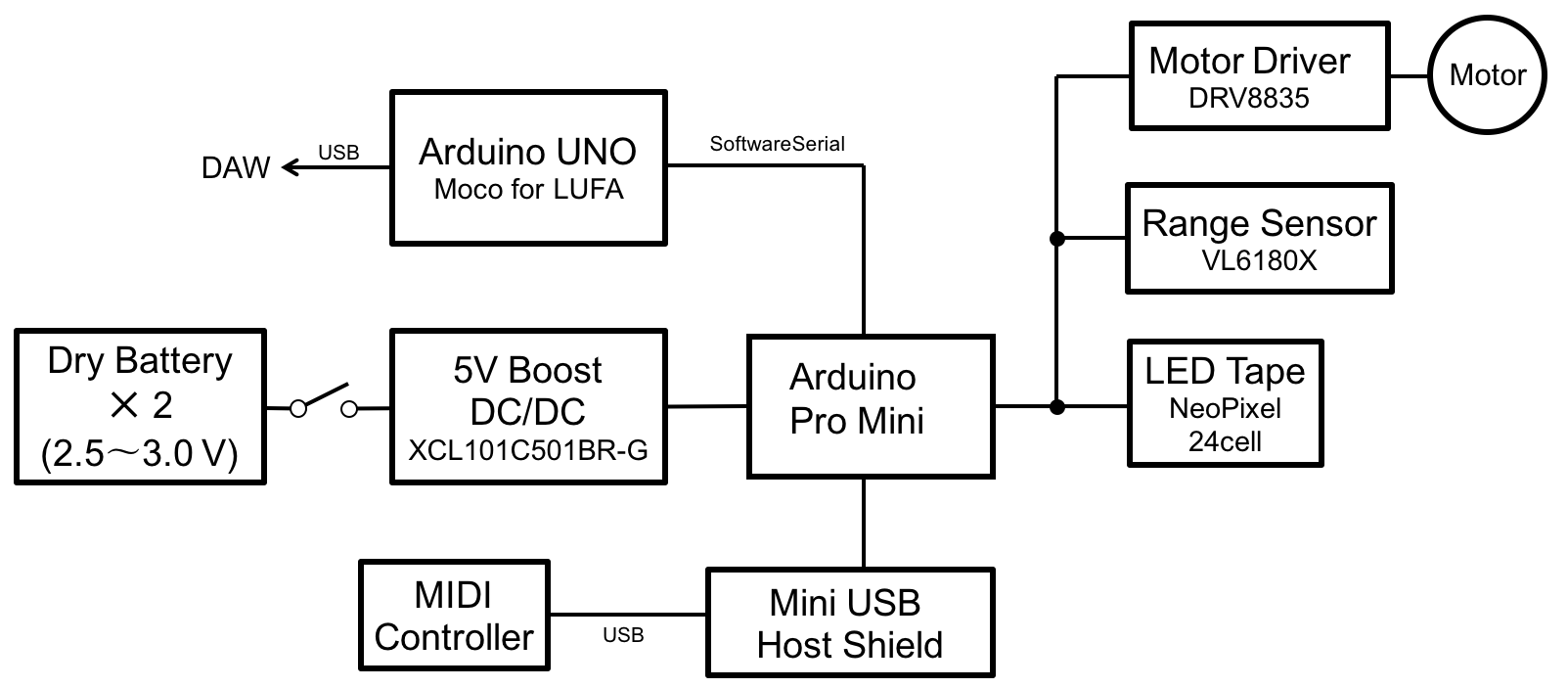
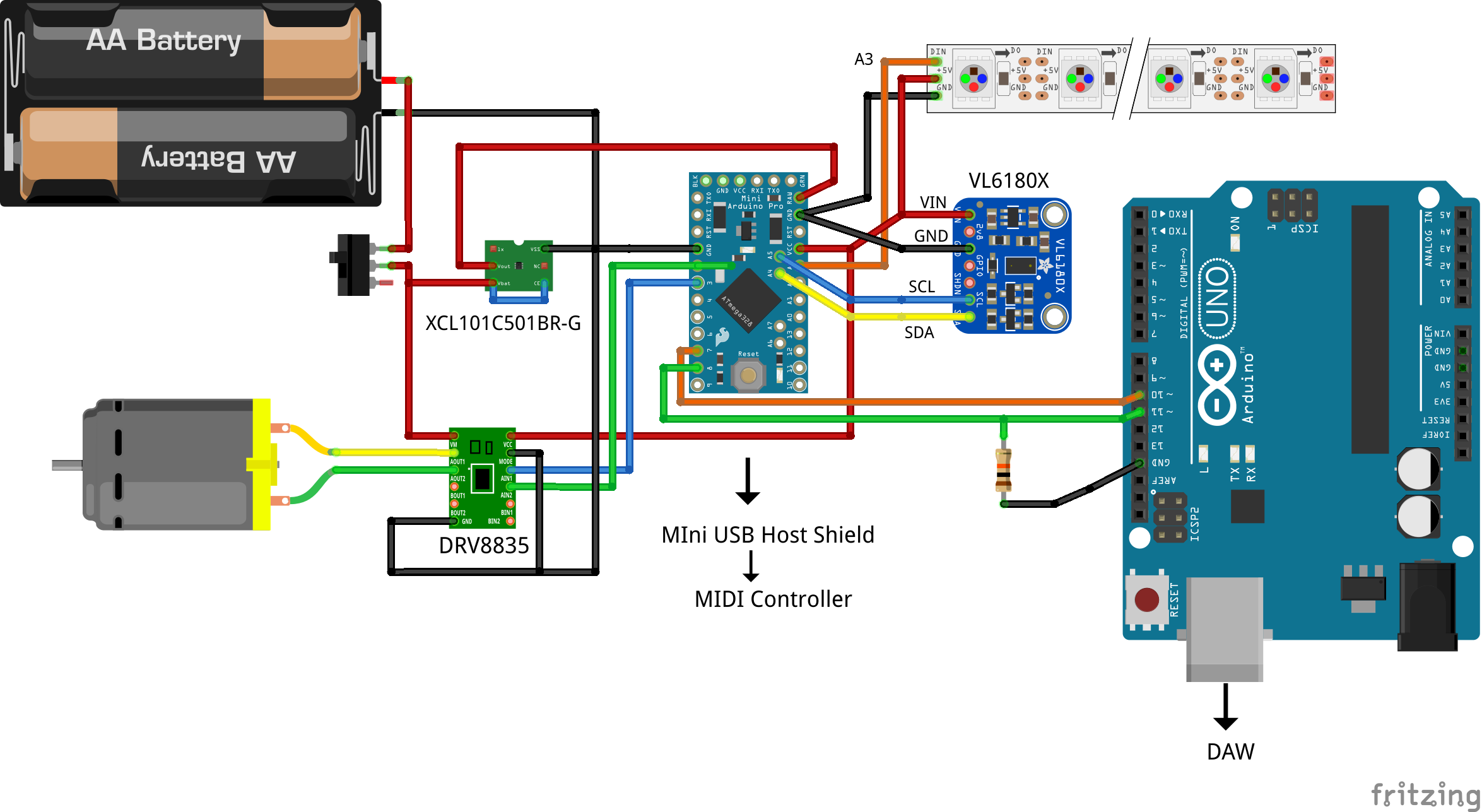
MEMIDION is a slide whistle that controlled by the micro-controller. The motor with the caterpillar slides the slide whistle. The slide amount is controlled by ToF range finder sensor VL6180. LED Tape NeoPixel emit light following the amount of slide.
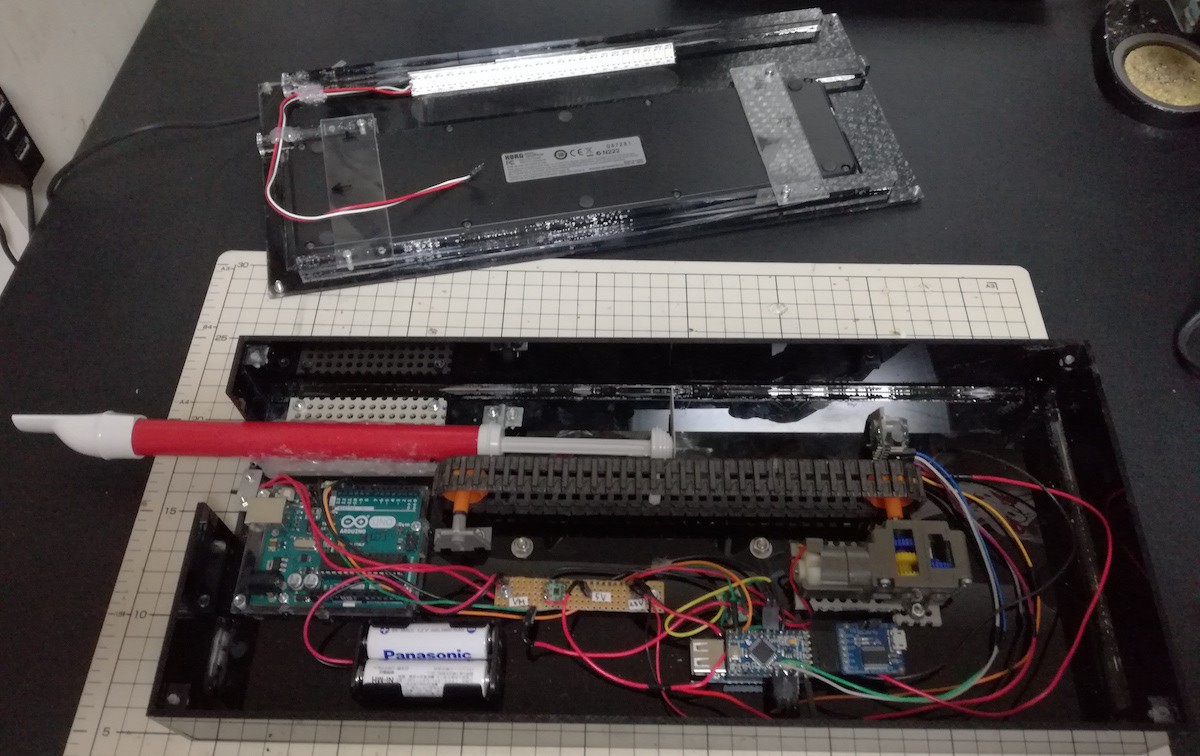
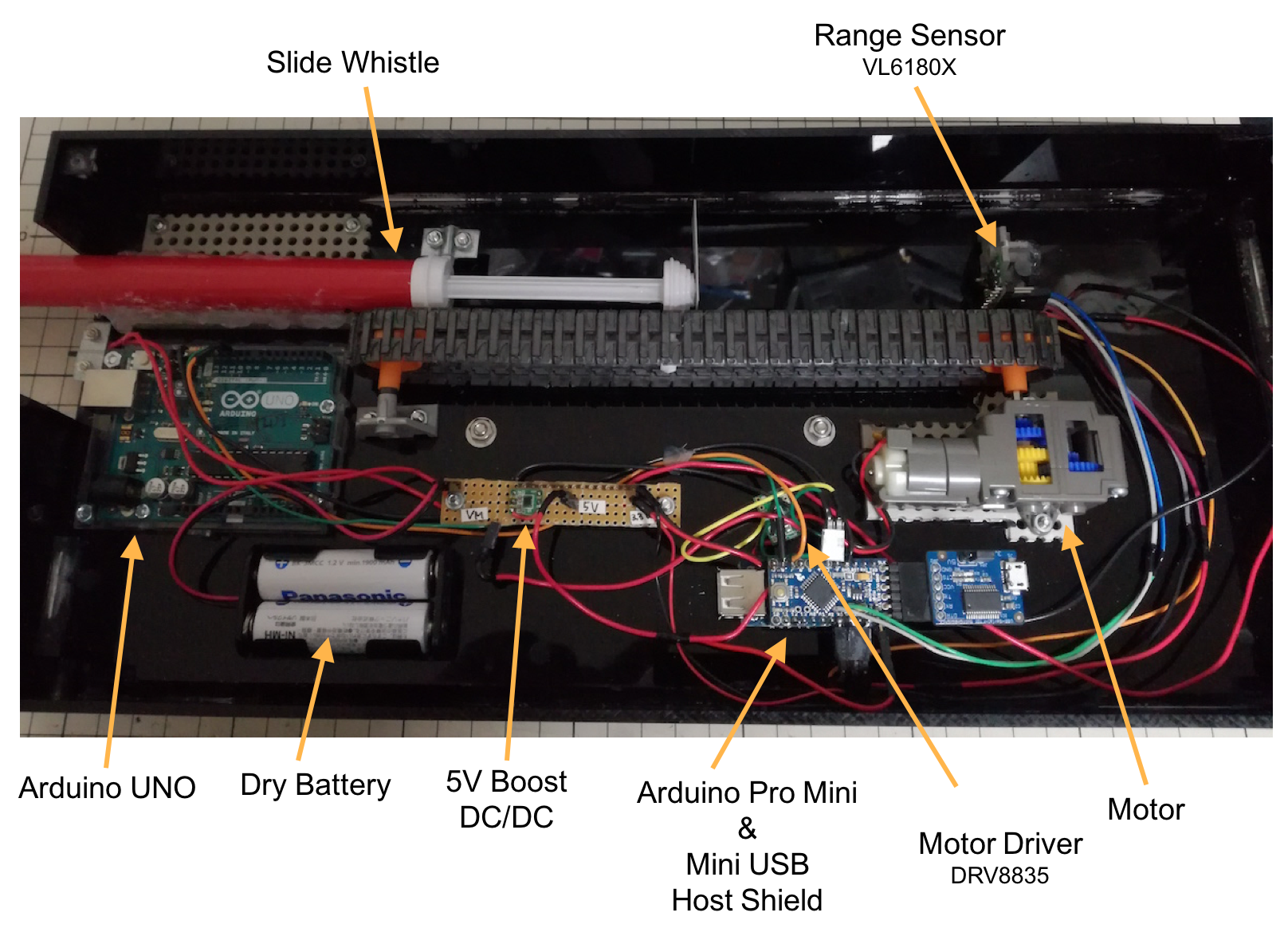
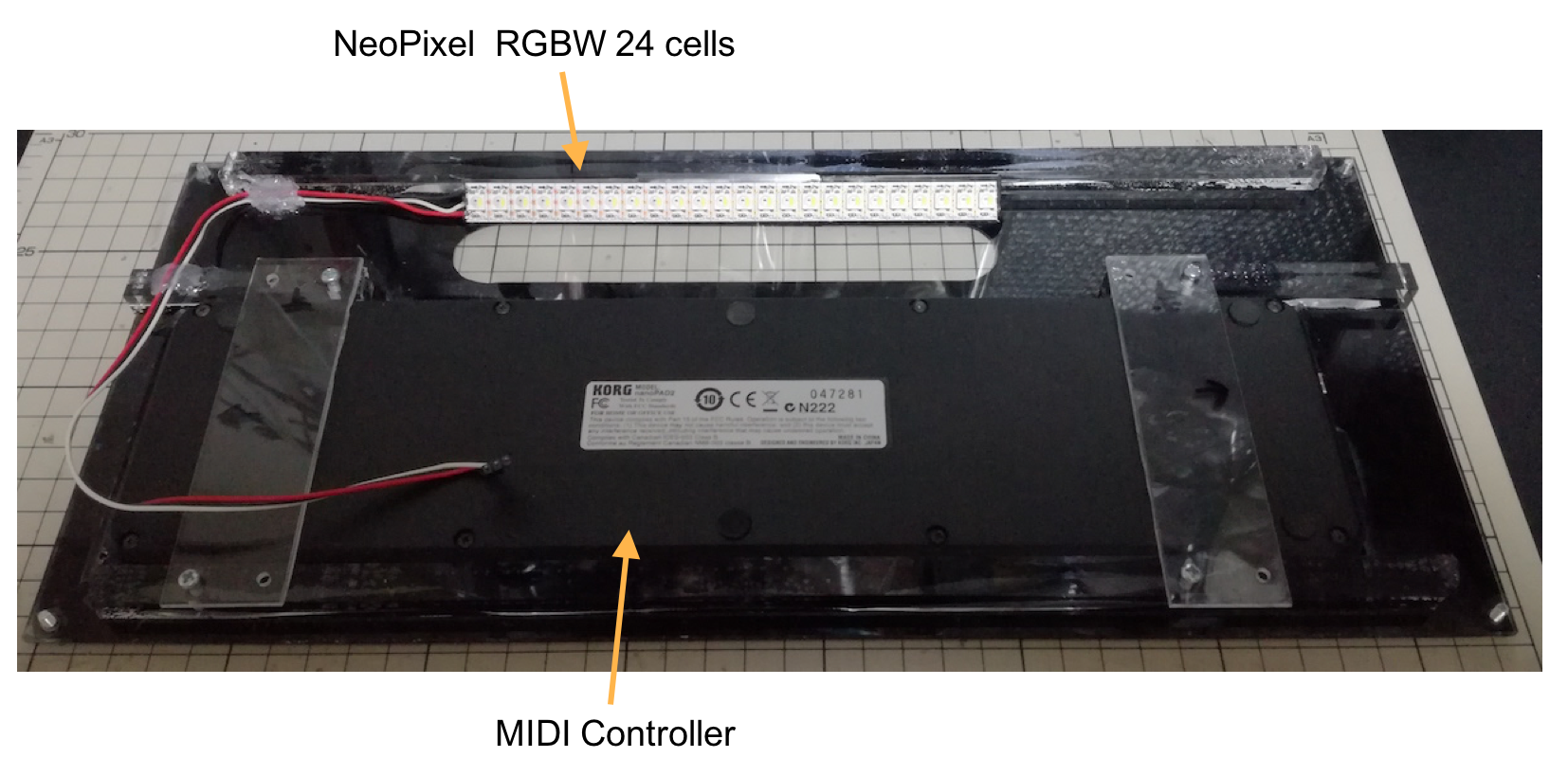
DAW Sound Source Mode
The MEMIDION works in two modes, DAW Sound Source Mode and Instrument Mode.
When PC (DAW) is connected to Arduino UNO, it switches to DAW Sound Source Mode.
Send a MIDI signal from the DAW and move MEMIDION.
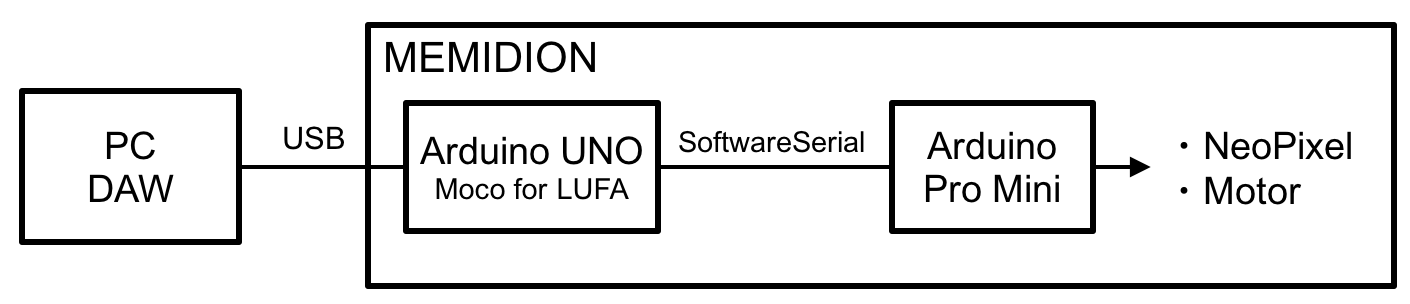
Arduino USB-MIDI device conversion
Write Moco for LUFA firmware to Arduino and let Arduino recognize it as a MIDI device.
The detail is right below.
https://github.com/kuwatay/mocolufa
Arduino UNO receives MIDI signals and sends them to Arduino Pro Mini with SoftwareSerial to control the motor and NeoPixels.
Setting of DAW software Reason
Anything is OK if DAW can output MIDI, I used Reason this time.
Place the external MIDI instrument and select "MocoLUFA" for the device.
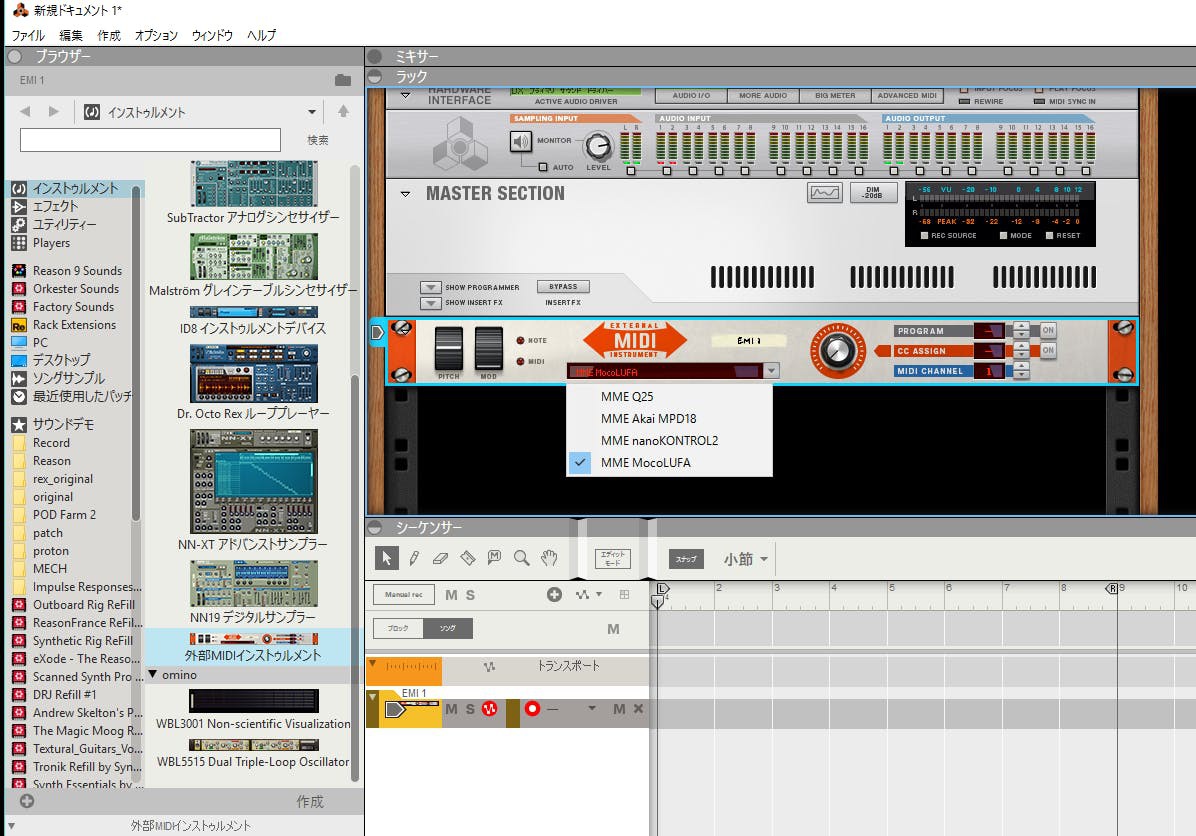
Operation
Automatic performance as MIDI sound source with DAW is also possible. My breath has to keep blowing....
Instrument Mode
When MEMIDION is not connected to the PC (DAW), it operates as a single musical instrument.
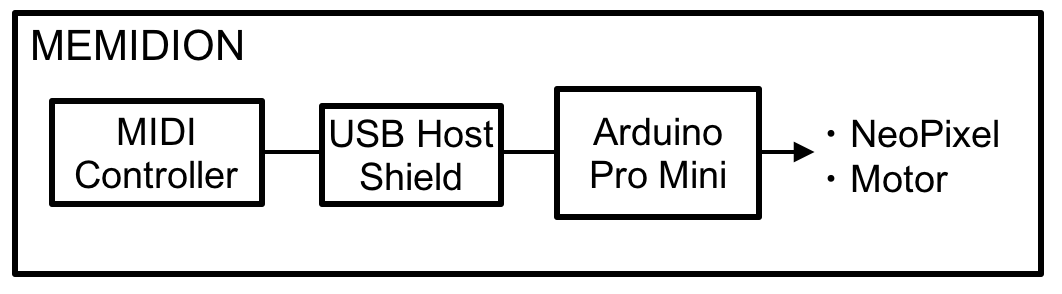
It receives MIDI signal from MIDIController via USB Host Shield and controls the motor and NeoPixels with Arduino Pro Mini.
Operation
Arduino IDE Code
I used the following as a USB MIDI library
https://github.com/felis/USB_Host_Shield_2.0
I programmed with reference to the following
https://github.com/felis/USB_Host_Shield_2.0/tree/master/examples/USBH_MIDI/USBH_MIDI_dump
The library for distance sensor VL6180X is below.
https://github.com/pololu/vl6180x-arduino
The library for NeoPixel is below.
https://github.com/adafruit/Adafruit_NeoPixel
#include <usbh_midi.h>
#include <usbhub.h>
#include <SPI.h>
#include <Wire.h>
#include <VL6180X.h>
#include <Adafruit_NeoPixel.h>
#include <SoftwareSerial.h>
SoftwareSerial mySerial(7, 9); // RX, TX
USB Usb;
USBH_MIDI Midi(&Usb);
uint8_t Note, oldNote;
VL6180X sensor;
int meas;
int aim = 50;
int diff = 6;
#define PIN A3
#define NUM_LEDS 24
#define BRIGHTNESS 30
int num;
int modeState = 0;
Adafruit_NeoPixel strip = Adafruit_NeoPixel(NUM_LEDS, PIN, NEO_GRBW + NEO_KHZ800);
void setup() {
Serial.begin(115200);
mySerial.begin(9600);
pinMode(8, INPUT);
Wire.begin();
pinMode(2, OUTPUT);
pinMode(3, OUTPUT);
digitalWrite(2, LOW);
digitalWrite(3, LOW);
sensor.init();
sensor.configureDefault();
sensor.setTimeout(500);
strip.setBrightness(BRIGHTNESS);
strip.begin();
strip.show();
if (Usb.Init() == -1) {
while (1); //halt
}
delay( 200 );
}
void loop() {
if(modeState != digitalRead(8)){
modeState = digitalRead(8);
Serial.print("Mode : ");
Serial.println(modeState);
}
//Instrument Mode
Usb.Task();
if (Usb.getUsbTaskState() == USB_STATE_RUNNING && modeState...
Read more »