/**
* The ALU (Arithmetic Logic Unit).
* Computes one of the following functions:
* x+y, x-y, y-x, 0, 1, -1, x, y, -x, -y, !x, !y,
* x+1, y+1, x-1, y-1, x&y, x|y on two 16-bit inputs,
* according to 6 input bits denoted zx,nx,zy,ny,f,no.
* In addition, the ALU computes two 1-bit outputs:
* if the ALU output == 0, zr is set to 1; otherwise zr is set to 0;
* if the ALU output < 0, ng is set to 1; otherwise ng is set to 0.
*/
// Implementation: the ALU logic manipulates the x and y inputs
// and operates on the resulting values, as follows:
// if (zx == 1) set x = 0 // 16-bit constant
// if (nx == 1) set x = !x // bitwise not
// if (zy == 1) set y = 0 // 16-bit constant
// if (ny == 1) set y = !y // bitwise not
// if (f == 1) set out = x + y // integer 2's complement addition
// if (f == 0) set out = x & y // bitwise and
// if (no == 1) set out = !out // bitwise not
// if (out == 0) set zr = 1
// if (out < 0) set ng = 1
CHIP ALU {
IN
x[16], y[16], // 16-bit inputs
zx, // zero the x input?
nx, // negate the x input?
zy, // zero the y input?
ny, // negate the y input?
f, // compute out = x + y (if 1) or x & y (if 0)
no; // negate the out output?
OUT
out[16], // 16-bit output
zr, // 1 if (out == 0), 0 otherwise
ng; // 1 if (out < 0), 0 otherwise
PARTS:
// zx
And16(a=x, b=false, out=zxTrue);
Mux16(a=x, b=zxTrue, sel=zx, out=zerox);
// nx
Not16(in=zerox, out=notx);
Mux16(a=zerox, b=notx, sel=nx, out=negx);
// zy
And16(a=y, b=false, out=zyTrue);
Mux16(a=y, b=zyTrue, sel=zy, out=zeroy);
// ny
Not16(in=zeroy, out=noty);
Mux16(a=zeroy, b=noty, sel=ny, out=negy);
// f
And16(a=negx, b=negy, out=andfxy);
Add16(a=negx, b=negy, out=addfxy);
Mux16(a=andfxy, b=addfxy, sel=f, out=fxy);
// no
Not16(in=fxy, out=notfxy);
Mux16(a=fxy, b=notfxy, sel=no, out=out);
}
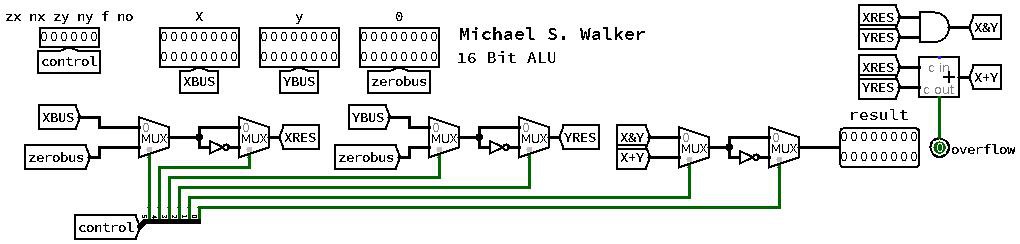
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.