Last time, I successfully made a python script that made a single component, but that made several assumptions, first, i was already in the footprint editor with a named component. if i was going to make ~200 components i would need to open many new footprints and name them in an intelligent way.
NAMING
From the Datasheet
All of these blank spots above can be filled in to form valid part numbers. only some of these cause changes to the footprints.
- the number of positions
- the termination type
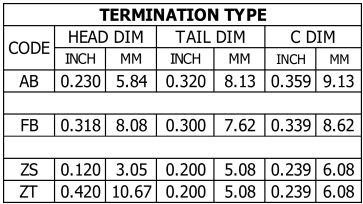
So, part numbers can be represented as "***CnnnSttC-MR30RC" where
- * is dont care
- n is number of pins
- tt is termination type
That is enough to make unique package names.
nameTemplate = "XX*C0{0:02d}{1:s}C-M30RC"
terminationTypes={'AB':.359,'FB':.339,'ZS':.239,'ZT':.239}
for Key,Val in terminationTypes.items():
for p in range(2,20+1):
myCMDs.append("remove '"+nameTemplate.format(p,Key)+".pac'")
myCMDs.append("EDIT '"+nameTemplate.format(p,Key)+".pac'")
The EAGLE "EDIT" command will create or open a package,symbol, or device from an open library. you can provide the suffix .pac, .dev, or .sym to explicitly create new items in the library. The remove command is only needed if you are running this script multiple times to debug it. you cant add duplicate pads to an existing footprint, so we just delete it first.
I've also created a quick dictionary with the measurements for each termination type. it may seem redundant to include both "ZS" and "ZT" variants, they have the same measurements as far as the footprints are concerned. however, i use EagleUp 3d visualization heavily, so its worth it to specify the two variants for later 3d modeling, and since I'm scripting everything, it doesn't really cost me anything either.
The nested loops above will create footprints for all four variants, each for two to twenty pins.
Here is everything combined, first in pseudo code:
for each variant, v
for each pin count p
create footprint(v,p)
for 1 to p
place SMD pad
draw documentation
And the full python:
hpitch = 0.1
nameTemplate = "XX*C0{0:02d}{1:s}C-M30RC"
cmdTemplate = "SMD {0:f} {1:f} '{2:s}' ({3:f} {4:f});"
cmd1Template = "WIRE .008 ({0:f} {1:f}) ({2:f} {3:f}) ({0:f} {1:f}) ;"
myCMDs=[]
terminationTypes={'AB':.359,'FB':.339,'ZS':.239,'ZT':.239}
for Key,Val in terminationTypes.items():
outer=Val
padW=.039
padH=outer/2
yPrime = +(padH/2)
yAlt = -(padH/2)
for p in range(2,20+1):
myCMDs.append("remove '"+nameTemplate.format(p,Key)+".pac'")
myCMDs.append("EDIT '"+nameTemplate.format(p,Key)+".pac'")
myCMDs.append("GRID 0.05 inch;")
x=0
for i in range(1,p+1):
if i%2==1:
y=yPrime
else:
y=yAlt
myCMDs.append(cmdTemplate.format(padW,padH,str(i),x,y))
x+=hpitch
myCMDs.append("LAYER tDocu;")
myCMDs.append("Set Wire_Bend 0;")
myCMDs.append(cmd1Template.format(-.05,0.05,(p)*.1-0.05,-0.05))
myCMDs.append("Set Wire_Bend 4;")
myCMDs.append(cmd1Template.format(-.05,0.05,(p)*.1-0.05,-0.05))
myCMDs.append("write")
Up to this point I was writing the python in notepad++, copying everything, using the %paste macro in ipython, then printing all the lines, copying them out of the command prompt, pasting them into a temporary file, then running that file in eagle. that got old exactly this fast.
So a quick addendum to the previous python:
with open("test.scr","wt") as f:
for x in myCMDs:
f.write(x+"\r\n")
After some quick cleanup and checking, I am now the proud owner of 200 clean footprints....
how do i connect 200 footprints to their respective devices?
more later.
Discussions
Become a Hackaday.io Member
Create an account to leave a comment. Already have an account? Log In.