Fitting a Quadratice Bezier Curve to Digitised Data
Here is the problem image:
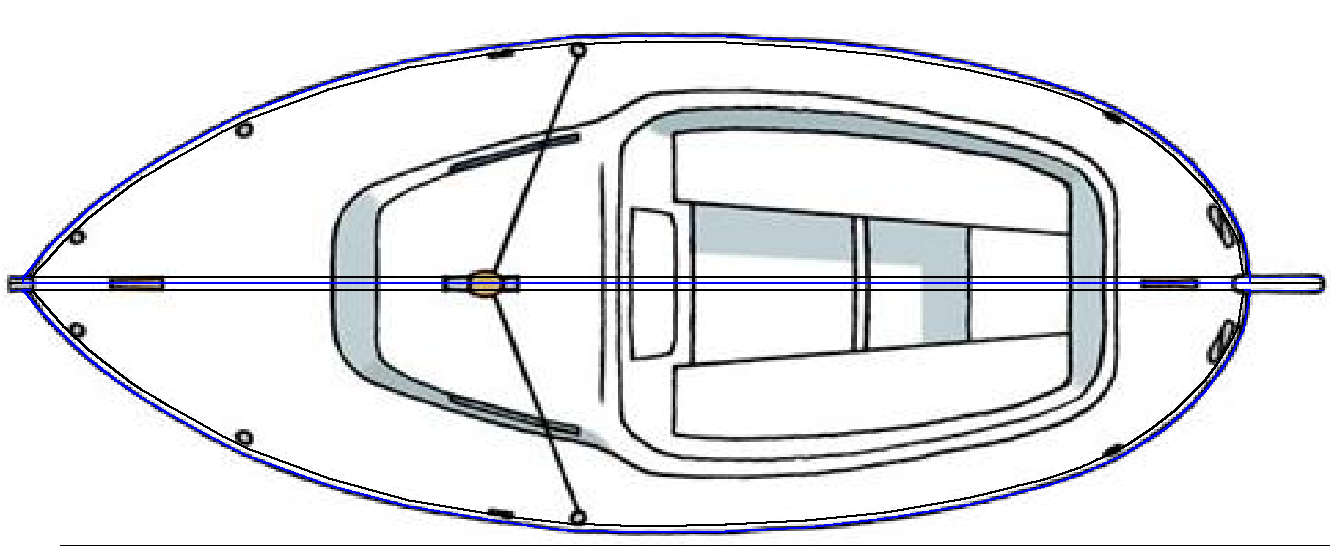
(source: https://www.oostzeejol.de/index.php/en/international-2/lynaes-14)
The blue and black digitised lines are the Gunwale and Sheer (beam) of the sailboat design I want to reverse engineer. The image is a plan of the Lynaes 14 sailboat that I found on the Internet.
I have previously tried to model the beam in Excel using polynomial curve fitting but the results were not satisfactory.
However, fitting quadratic Bezier curves was excellent:
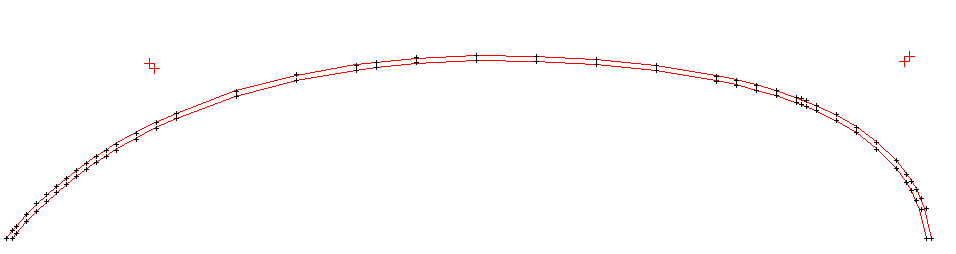
The image has four Bezier curves joined at the max beam.
Note how well the Bezier curves meet at the stern. This is a problem area for polynomial curves.
Here is the polynomial 6th order fit. While it looks okay at this scale, the beam wanders and the curve at the stern is not ideal (i.e. perpendicular):
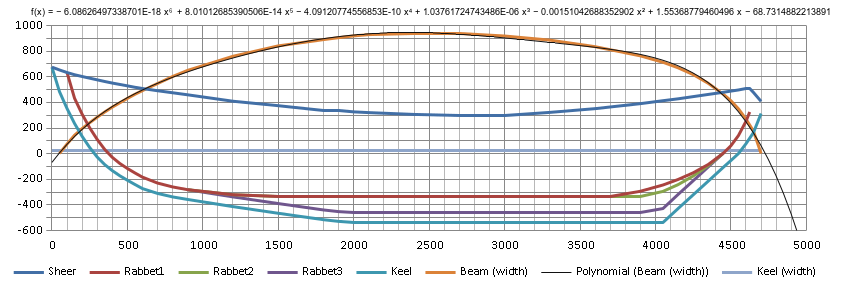
Here is an image of a Lynaes 14 stern which is near perpendular to the keel:
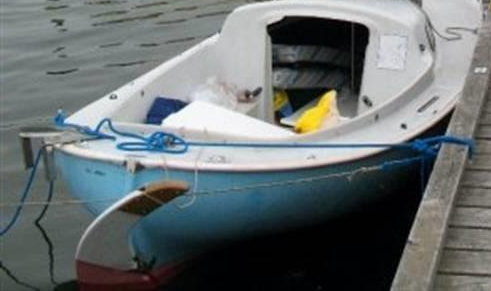
(source: https://www.baadgalleri.dk/galleri/billeder/lynaes-14/5243)